Table of content
HI!! Kai is here
This place is used to store and share the knowledge about programming. The followings are the topic which I am learning currently:
Feel free to share your thoughts, and let's improve together.
Note ↵
Angular ↵
Angular#
Angular is a JavaScript Framework which allows you to create reactive Single-Page-Application (SPA), and TypeScript as its programming language.
Introduction#
Comparison#
優勢 | 劣勢 | 機會 | 威脅 | |
---|---|---|---|---|
Angular | 在企業及項目中有強大的市場份額,適合開發大型、複雜的應用程式 | 強大的工具組合,提供了豐富的功能和模組化的架構 | 學習曲線較陡峭,對於初學者來說可能需要更多時間去理解和掌握 | 隨著React和Vue的快速崛起,可能會面臨更多競爭和市場份額下降的壓力 |
React | 適用於快速原型驗證和敏捷開發,在前端領域仍然具有很高的成長空間 | 擁有龐大而活躍的社群支持,提供了高效的虛擬dom和組件化開發方式 | 相比其他框架,react本身只是一個視圖層庫,需要額外整合其他庫才能構建完整應用程式 | 因為生態系統龐大而不斷增長,可能會出現版本更新和庫相容性問題 |
Vue | 作為輕量級框架,易於集成到現有項目中,並且在中小型應用程式開發中具有潛力 | 簡單易學且輕量級,具有良好的文件資源和友好的生態系統 | 社群規模相對較小,在某些方面可能缺乏廣泛的支持和資源 | 視為新興框架,可能需要更多時間才能贏得企業級項目的信任和選擇 |
Development#
- Angular 1 跟之後的版本差異很大,因此稱作 AngularJS,而之後的稱作Angular
- Angular 2 是 Angular 1 重新編寫完成的,3被跳過,而之後每六個月出一次新的版本
Bootstrapping#
graph LR
A[Index.html] --> B[Main.ts];
B --> C[App.modules.ts];
C --> D[App.component.ts];
D --> E[App.component.html];
Angular 專案架構#
檔案 | 用途 |
---|---|
node_modules | 放置相關 Angular 的套件 |
.editorconfig | 程式碼編輯器的設定 |
angular.json | 工作區中所有CLI項目的配置 |
package-lock.json | Node_module npm 客戶端所需的配件及版本 |
package.json | 列出依存項目 |
tsconfig.json | TS相關配置 |
component | 應用程式主要的邏輯及頁面範本、樣式 |
assets | 圖檔、樣式、JS… |
index.html | 應用程式的首頁 |
main.ts | 應用程式主要的入口點 |
Module and @ngModule#
目的#
- 將巨大的應用程式拆成一個一個模塊
- 每個模塊都包含特定功能的函式庫或元素
JS模組#
- export: 此程式可用於其他模組
- import: 引入其他模組到此程式
- Angular 框架採用
Angular模組#
- 使用 @ngModule 定義 metadata 供編譯使用
@ngModule#
- declarations: 模組中可宣告的物件,像是Component、Directive、Pipe,是這個模組所擁有的元件
- imports: 要使用外部模組,必須先引入到這個模組才可以使用外部模組的物件
- providers: 加入服務提供者,會以執行階段動態注射的方式載入
- bootstrap: 指定Angular入口元件,Angular會建立這個元件
常用模組#
Module | 用途 |
---|---|
BrowserModule | 在瀏覽器執行應用程式時 |
CommonModule | 使用ngIf、ngFor |
FormsModule | 使用 ngModel 及 template driven form 時 |
ReactiveFormsModule | 使用 Reactive Form |
RouterModule | 使用 router 功能 |
HttpClientModule | 與 遠端伺服器 連結時需要用到 |
Lifecycle#
stateDiagram-v2
[constructor] --> [ngOnChanges]
[ngOnChanges] --> [ngOnInit]
[ngOnInit] --> [ngDoCheck]
[ngDoCheck] --> [ngAfterContentInit]
[ngAfterContentInit] --> [ngAfterContentChecked]
[ngAfterContentChecked] --> [ngAfterViewInit]
[ngAfterViewInit] --> [ngAfterViewChecked]
[ngAfterViewChecked] --> [ngOnDestroy]
- ngOnChanges
-
- Called after a bound input property changes
-
- 當綁定的值變動時觸發,可觸發多次
- ngOnInit
-
- Called once the component is initialized
-
- 在onChanges執行完後觸發,若onChanges沒有觸發則直接觸發,只觸發一次
- ngDoCheck
-
- Called during every change detection run
- ngAfterContentInit
-
- Called after content (ng-content) has been projected into view
-
<ng-content>
被呼叫後觸發,只觸發一次
- ngAfterContentChecked
-
- Called every time the projected content has been checked
-
- doCheck之後調用
- ngAfterViewInit
-
- Called after the component’s view (and child views) has been initialized
-
- 各種UI被組裝完成後調用,只調用一次 EX:
<input>
標籤載入
- 各種UI被組裝完成後調用,只調用一次 EX:
- ngAfterViewChecked
-
- Called every time the view (and child views) have been checked
-
- EX:
<input [(ngModel)] = "test" >
當綁定值變更時被觸發
- EX:
- ngOnDestroy
-
- Called once the component is about to be destroyed
-
- 只觸發一次,通常會在onDestroy的地方取消訂閱,避免memory leak
語法#
- @Input
-
- 允許data流入子component
- @Output
-
- 允許子component往外傳送data
- @ViewChild
-
- We need @ViewChild if we want to access a template's local reference from the class of the same component (if we can't pass it as a method parameter).
- 可以直接讓parent component直接呼叫child內提供的public 屬性和方法,不需要使用 @Input和 @Output
- 需要在
ngAfterViewInit()
後才能使用 - 取得有兩種方式:
- #id
-
className
.html
.ts
- @ViewChildren
-
- 需要在
ngAfterViewInit()
後才能使用
- 需要在
- @ContentChild
-
- 需要在
ngAfterContentInit()
後才能使用
- 需要在
- @ContentChildren
-
- 需要在
ngAfterContentInit()
後才能使用
- 需要在
- @HostListener
-
- 提供元素事件的處理方法,可以綁定任何標準的DOM事件
ng-content
- ng-template
-
- Tag不會顯示在HTML上,透過參考才會呈現 → 被動的
- ng-container
-
- DOM的容器,Tag不會顯示在HTML上,只會單純顯示內容
Templates#
Binding#
DOM Property Binding#
- {{ value }} is called Interpolation
- Property Binding uses
[]
- also called source-to-view
- 舉例:
HTML Attribute Binding#
- 多數的 HTML Attribute 會對應到 DOM Property
- 某些 Attribute 只是單純的 Attribute
- ARIA、SVG、Table 跨行跨列的 span
- 舉例:
Class Binding#
-
CSS class 綁定也是一種屬性綁定
.ts
.html
-
ngClasses
Event Binding#
- View to source
- 使用 (事件) 或是 on-事件
-
舉例
-
Bubbling (冒泡)
- 事件由內向外
- 舉例:
- 上面事件會先由button的event先觸發,再來才是div的
- 可以使用
stopPropagation()
來阻止冒泡
$event 事件處理訊息#
- 相當於DOM物件的event物件
- 綁定會通過這個傳遞關於此事件的資訊
- event 物件
- event.target: 原始目標 → 觸發的對象
- event.currentTarget: 當前這個目標 → 監聽的對象
- event.target.value
- 提供 DOM Event 的相關方法
- preventDefault → 取消事件預設行為
- stopPropagation → 取消事件傳遞行為
- stopImmediatePropagation → 馬上取消所有的事件傳遞行為
- DOM Level 3 新增的功能
- 下拉清單使用 $event.target
- 觸發事件的目標物件
[ngModel]、(ngModelChange)#
- 提供表單控制項的綁定能力
- 需要使用 NgModule、FormsModule
- [ngModel] → source to view → 數據綁定
- (ngModelChange) → view to source → 事件綁定
[(ngModel)]#
- 雙向綁定,view-to-source-to-view
- 只能用在HTML中
- @input → 宣告自訂元素的輸出屬性
- @output → 宣告自訂元素的是件通知
- EventEmitter → 定義事件的傳遞訊息型別
- 自訂的元素需要注意:
- @output 名稱必須是 @input + 事件名稱
- 舉例:
- EventEmitter 使用 emit 傳遞事件參數
Style Binding#
-
使用 [style.attribute]
-
有多個style要設定時,使用ngStyle
Pipe#
用來對於字串、日期、數字、貨幣等等資料進行轉換及格式化
內建:
Pipe | 用途 |
---|---|
UpperCasePipe | uppercase |
LowerCasePipe | lowercase |
DatePipe | date |
CurrencyPipe | currency |
DecimalPipe | number |
PercentPipe | percent |
Directives#
- components
- component and template
- structural directives
- 改變 view 的結構
- ngFor、ngIf
- attribute directives
- 改變 Element、component 或其他 directive 的外觀或行為
- ngStyle、ngClass
Structural Directives (結構型指令)#
- Angular 使用結構型指令操作DOM
- 具有重塑DOM結構的能力 → 新增、移除或是維護元素
-
使用 * 為字首
-
*ngFor: 重複顯示每一個單項
- 提供 index 屬性 → 以0為開始的索引
-
提供 boolean 屬性
- first、last: 首筆或是尾筆為 true
- evne、odd: 偶數或是基數時為 true
-
*ngIf: 顯示項目,條件不符整個元素不存在
-
*ngSwitch: 類似JS的switch,只會有一個被顯示
- 搭配
ngSwitchCase
和ngSwitchDefault
- 搭配
-
Routes#
Route 包含以下屬性#
- path
-
- 路徑
- 在route path當中使用 '**' → 以上皆非,所以要放在path最下面
- 可以定義參數,例如 → :id、:user
- component
-
- 符合路徑時要啟動的元件
- title
-
- 頁面的名字
- data
-
- 可以自訂額外的資料,也可以不傳遞
- redirectTo
-
- 重新導向的URL
- path 預設空字串
- pathMatch 必須指定此屬性
- full 確保路徑完整符合URL
- 預設是 prefix ,路徑由左檢查只要部分符合就會套用
- outlet
-
- 放在對應的routerOutlet
- children
-
- 子路由的 Routes 物件,指定巢狀路由
routerLink#
<a routerLink = 'page1'></a>
<a [routerLink] = "[ '../', 'page1' ]"></a>
<a [routerLink] = "[ '/', 'customer', 'Company1' ]"> </a>
- 使用
/
為絕對路徑,會從根路由開始找 - 使用
./
為相對路徑,會從當前路由的子結點路徑開始找 - 使用
../
會從上一級結點找 → 相對父路徑
routerLinkActive#
- 一個屬性指令,在賦予值的時候須加上單引號,否則angular會認為是變量
- 在路由active時可以添加class在DOM元素上,url變化時才會移除
- Router.navigate()
- 以
[]
路徑陣列傳遞參數
- 以
- Router.navigateByUrl()
- 以路徑字串傳遞
接收參數#
-
使用 ActiveRoute 的 snapshot
-
在 ngDoCheck() 獲取參數值
Form#
Form#
Reactive Form | Template-driven Form | |
---|---|---|
建立表單模型 | 明確的在 component 建立 FromControl | 隱含式,使用 directive 在 template中 |
資料模型 | 結構化、不可變 | 非結構化、可變 |
資料流 | 同步 | 非同步 |
表單驗證 | 函式 | 指令 |
Reactive Form#
- 可擴展性
- 可重用性
- 可測試性
- 相關 Directive
- FormControlDirective
- FormControlName
- FormGroupDirective
- FormGroupName
- FormArrayName
-
需要實體化值與狀態
.ts
.html
-
要建立FormGroup的實體 .ts
.html
-
FormControl 存取值
- setValue()
- 適用單個FormControl更換新值
- pathValue()
- 可指定替換特定屬性的值
- get(’key’).value
- 取得指定的 key 的 FormControl 值
- setValue()
- 引用 @angular/forms 的 ReactiveFormsModule
Template-Driven Form#
- 簡單容易
- 擴展能力低
- 使用ngModel建立及管理FromControl賦予的表單元素
-
只需要定義變數
.ts
.html -
引用 @angular/forms 的 FormsModule
- novalidate
- 避免預設的UI
- ngNativeValidate
- 開啟預設的UI
ngModel追蹤輸入項目和驗證能力#
- 點擊過了?
- Y: ng-touched
- N: ng-untouched
- 變更了?
- Y: ng-dirty
- N: ng-pristine
- 驗證通過了?
- Y: ng-valid
- N: ng-invalid
- 使用
#
命名並指向 - 需搭配[(ngModel)]
ngForm#
- 聯繫到表單
- FormGroup 最上層
- @Output
- ngSubmit: 按下 submit 按鈕時的事件
- @Input
- control: FormGroup 的實體
- controls{[key: string]: AbstractControl} 控制項的對應
共用的基礎元件#
AbstractControl → 提供所有控制元件和某些共享的行為,像是
validate、FormControl、FormGroup、FormArray
- FormControl
- 管理單一輸入項(input、select)的值及輸入驗證狀態
- FormGroup
- 管理一組輸入項(FormControl、FormGroup、FormArray) 的值及輸入驗證狀態
- FormArray
- 管理輸入項陣列的值及輸入驗證狀態
- FormBuilder
- 用來建立 FormControl 的方法
表單驗證#
- 確認輸入正確
- 降低因輸入錯誤的網路往返
- 降低 server 不必要的負擔
哪些要驗證#
- 必要欄位
- 輸入值必須在特定範圍
- 輸入值必須符合格式
- 長度限制
HTML5基本驗證#
HTML | Angular |
---|---|
required | |
minlength、maxlength | |
pattern | |
min、max |
errors: 驗證錯誤的訊息#
- required: 若為空白則為 true
- minlength、maxlength: 不符合則為 true
- pattern: 使用 RegEx 驗算,不符合則為 true
- email: 不符合則為 true
ngModelGroup#
- 上層為ngForm
- 可以用來檢驗一組相關資料
NG_VALIDATORS#
- 自訂驗證的必須要註冊providers
- multi 必須設定為 true
- 在 @Directive 指定 providers 資訊
Service & HttpClient#
Service#
- Angular核心元件
- 專門處理某事情
- component → 畫面、資料綁定
- service → 取得資料、邏輯處理
- 模組化、增加重用性
- component 使用 Dependency Injection (依存注入) Service
- 通常 component 會共用一個 Service
- Service 的裝飾詞
- @Injectable
Service 註冊在#
-
@Injectable 等級
- 在 service
- 'root' 範圍在整個 app
-
@ngModule 等級
- 在 app.module
- 範圍在當前 module
-
@component 等級
- 在 .component.ts
- 範圍在當前 component
HttpClient#
- 以 Http 協定與後端服務進行通訊
- 引用 @angular/common/http 的 HttpClientModule
- 以 DI 方式帶入 HttpClient
Http 方法#
get(url: string, options)
post(url: string, body: any, options)
put(url: string, body: any, options)
delete(url: string, options)
- url : 目的端網址
-
options : 最常用 observe 與 responseType
-
observe 指定要回傳的內容
- body | events | response
-
responseType 指定格式
-
headers 指定標頭資訊
-
-
body : 要放在 HTTP Body 的資料
- 回傳 Observable 或是 Observable<
T
>
Observable#
- 可觀察物件傳遞 HttpClient 的回傳值
- 通常用於非同步設計及事件處理
- 使用 subscribe() 方法並傳入一個 observer
- observer 物件定義回呼叫函式
- next: 必要當處理的值送達時值行,可值行0-n次
- error: 可省略,處理錯誤
- complete: 可省略,處理執行完畢時的通知
- See more detail in: RxJS
RESTful Service#
- REST → Representational State Transfer
- 在不同架構、網路互相傳遞資訊
- 使用 HTTP 協定時做資料交換的一致性
- 基於 HTTP、URI、XML、HTML、JSON
- 對應HTTP協定的GET、POST、PUT、DELETE
- 以資源(URI)為基礎
ASP.Net Web API#
- ASP.NET Core MVC的一部分
- 是REST-style AP
- 使用URL提出請求
- 讓存取程式變得更簡單易寫
- 回應資料支援JSON格式
- 資源存取的邏輯重複使用
- Web API 的控制器由 ControllerBase 類別繼承
-
提供的方法
方法 狀態碼 OK() 200 BadRequest() 400 NotFound() 404 PhysicalFile() 回傳檔案
回應的型別#
-
IActionResult
- 回應多種類型
-
ActionResult
- Core 2.1 之後引進
- 透過泛型指定回應型別
CORS#
- 預防網頁不同網域提出需求
- 伺服器端需要啟用
Command#
-
Setup
Command Description npm install -g @angular/cli
install Angular CLI globally -
Create Application
Command Description ng new [name]
create new project ng new [name] --routing
create project with routing ng new [name] -s
will the style be in .ts file ng new [name] -S
will not generate spec file ng new [name] --create-application false
only create the workspace -
Generate
Command Description ng g app [name]
create new app in the project ng g c [name]
create component ng g s [name]
create service ng g cl [name]
create class ng g lib [name]
create library -
Build
Command Description ng serve
run the project ng serve -o
open app in the default browser ng serve --ssl
use the HTTPS ng serve --port [number]
use specific port ng serve build
build app to dist folder -
Other
Command Description ng version
check angular version
Ended: Angular
Git ↵
Git#
Git is a tool allows people to track any tiny footprint.
Commend Git Command#
Configuration#
Set name#
git config user.name "your name"
Set email#
git config user.email "your email"
Start#
init#
Create a new local repository in current directory
git init [project name]
clone#
Clone from exist remote repository
git clone [url]
Working#
status#
Show modified files
git status
log#
Show commit history
git log
add#
Add a file to staging area
git add [file] git add . # add all files
restore#
Restore the code
gir restore [file]
rm#
Let code move to untracked
git rm -cached [file]
diff#
Show changes between working directory and staging area
git diff [file]
commit#
Create a new commit for changed files in the staging area
git commit -m "your commit"
reset#
Remove file from the staging area
git reset [HEAD^]
reset the last commit
^
means go back one stage, if there is^^
, it means go back two stage if there is too many times, you can write it ascommit~3
orcommit^3
Different
mixed | soft | hard | |
---|---|---|---|
working directory | keep | keep | remove |
staging area | remove | keep | remove |
Caution
使用 --hard 的時候請注意!!
Storing#
stash#
Put current changes in your working directory into stash for later use
git stash
Different
Branching#
branch#
List all local branches in repository
git branch
checkout#
Switch working directory to the other branch
-
git checkout [-b]
-b: create the specified branch if it does not exist
merge#
Join specified branch into current branch
git merge [branch]
rebase#
Reapply commits on top of another base tip
Synchronizing#
fetch#
Fetch changes form the remote
git fetch [remote]
pull#
Fetch changes from the remote and merge current branch
git pull [remote]
- git pull = git fetch + git merge
push#
Push local changes to the remote
git push
remote#
- > Display the remote repository
Diagram#
sequenceDiagram
participant w as Workspace
participant sa as Staging Area
participant d as Branch develop
participant m as Local Repo
participant r as Remote Repo
participant c as Colleagues
rect
m ->> w: git init
r ->> w: git clone
end
w ->> sa: git add
sa ->> m: git commit
m ->> d: git checkout
d ->> m: git merge
Note over d, m: Merge request
m ->> r: git push
c ->> r: git push
rect
r ->> w: git pull
r ->> m: git fetch
m ->> w: git merge
end
m -) w: git reset --hard
m -) sa: git reset --soft
Note over w, sa: git reset --mixed
.gitignore#
GitHub & GitLab#
%%{
init: {
'logLevel': 'debug',
'theme': 'base',
'gitGraph': {'mainBranchOrder': 2 },
'themeVariables': {
'commitLabelColor': '#ffffff',
'commitLabelBackground': '#000000',
'commitLabelFontSize': '14px'
}
}
}%%
gitGraph
checkout main
commit id: "start"
commit id: "add feature1"
branch feature1 order: 3
commit id: "create feature1"
commit id: "pull request"
commit id: "code review"
checkout main
merge feature1 id: "MERGE1"
commit id: "add feature2"
branch feature2
commit id: "create feature2"
commit id: "pull request2"
commit id: "code review2"
checkout main
branch feature3 order: 4
commit id: "create feature3"
commit id: "fix error"
checkout main
merge feature2 id: "MERGE2"
commit
checkout feature3
commit id: "pull request3"
commit id: "code review3"
checkout main
merge feature3 id: "MERGE3"
commit
%%{
init: {
'logLevel': 'debug',
'theme': 'base',
'gitGraph': {'mainBranchOrder': 3, 'parallelCommits': false },
'themeVariables': {
'commitLabelColor': '#ffffff',
'commitLabelBackground': '#000000',
'commitLabelFontSize': '14px'
}
}
}%%
gitGraph
commit
branch pre-production order: 2
checkout pre-production
commit
branch production order: 1
checkout production
commit
checkout main
branch feature1 order: 4
checkout feature1
commit id: "merge request"
commit id: "code review"
checkout main
merge feature1 id: "MERGE1"
checkout pre-production
merge main
checkout production
merge pre-production tag: "1.0.0"
branch error1 order: 5
commit id: "merge request2"
commit id: "code review2"
checkout main
merge error1 id: "MERGE2"
checkout pre-production
merge main
checkout production
merge pre-production tag: "1.0.1"
Comparison#
GitHub flow | GitLab flow | |
---|---|---|
優點 |
|
|
缺點 |
|
|
References:#
Hands on#
Git Merge Conflict#
Resolve Git Merge Conflict
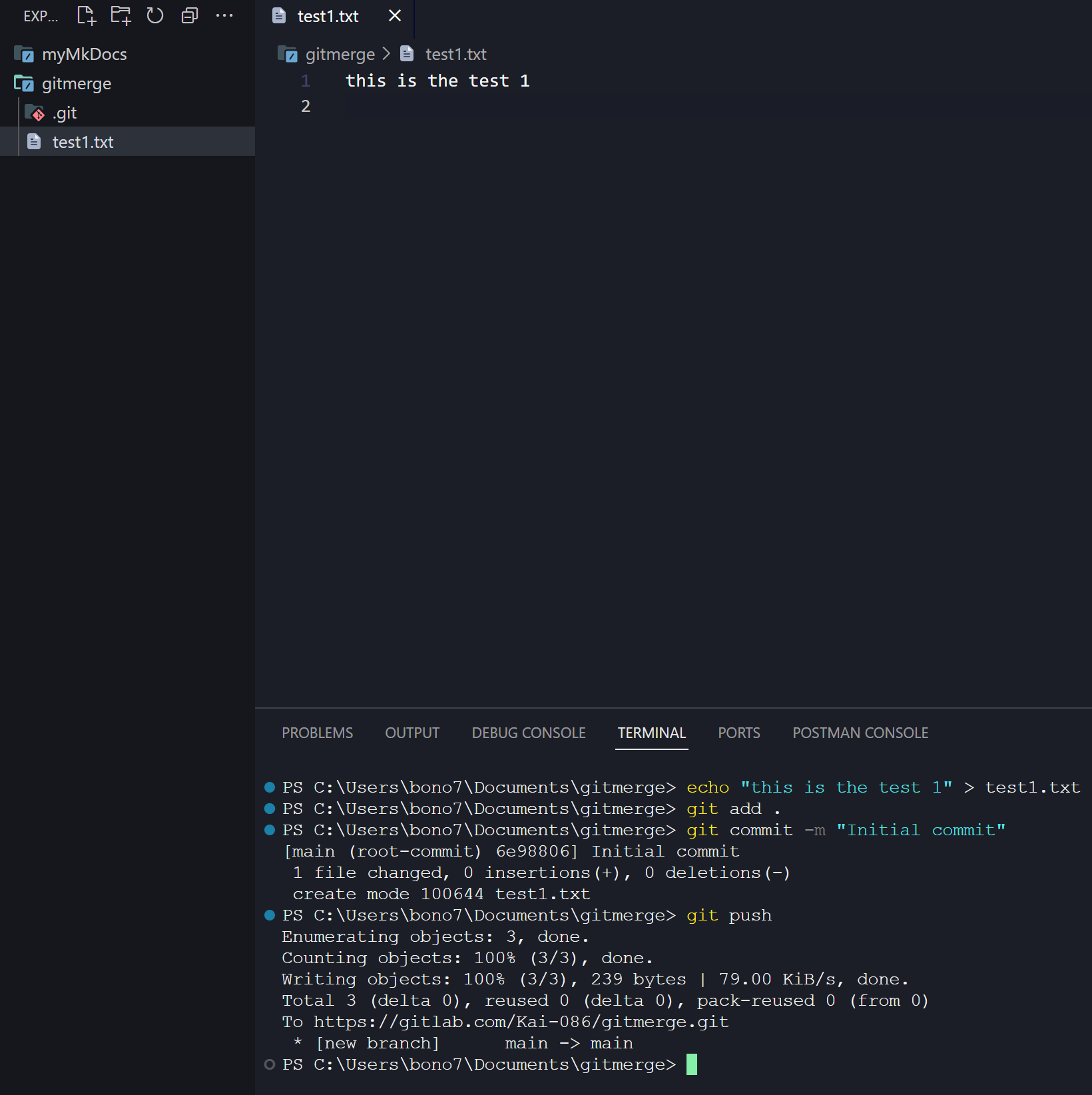
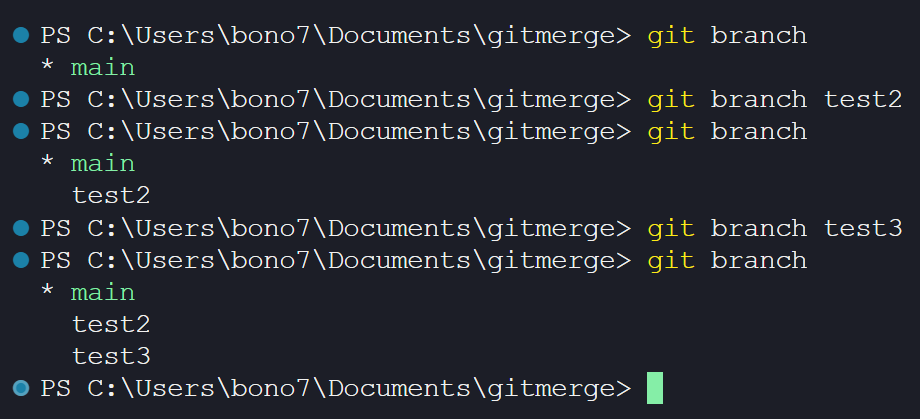
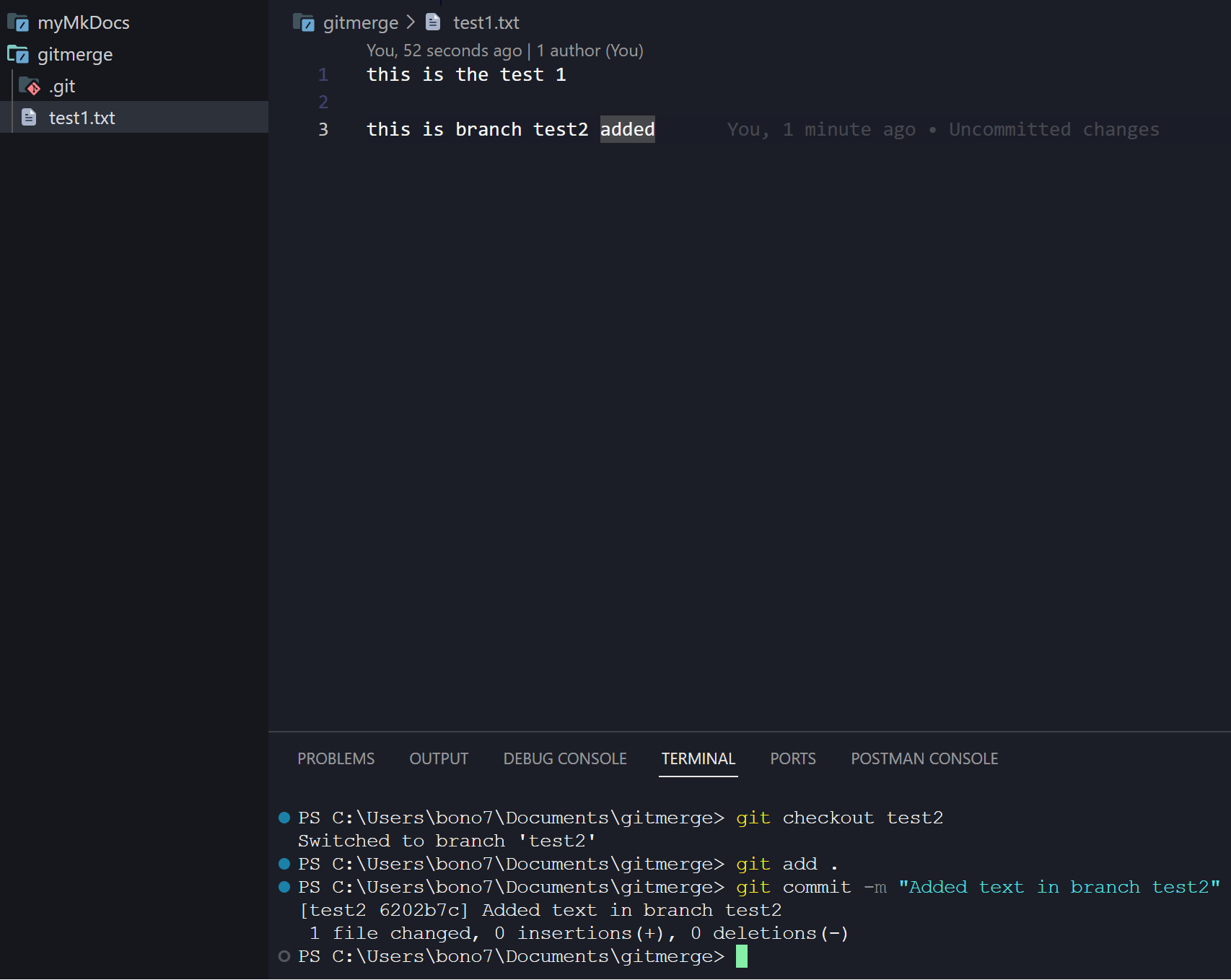
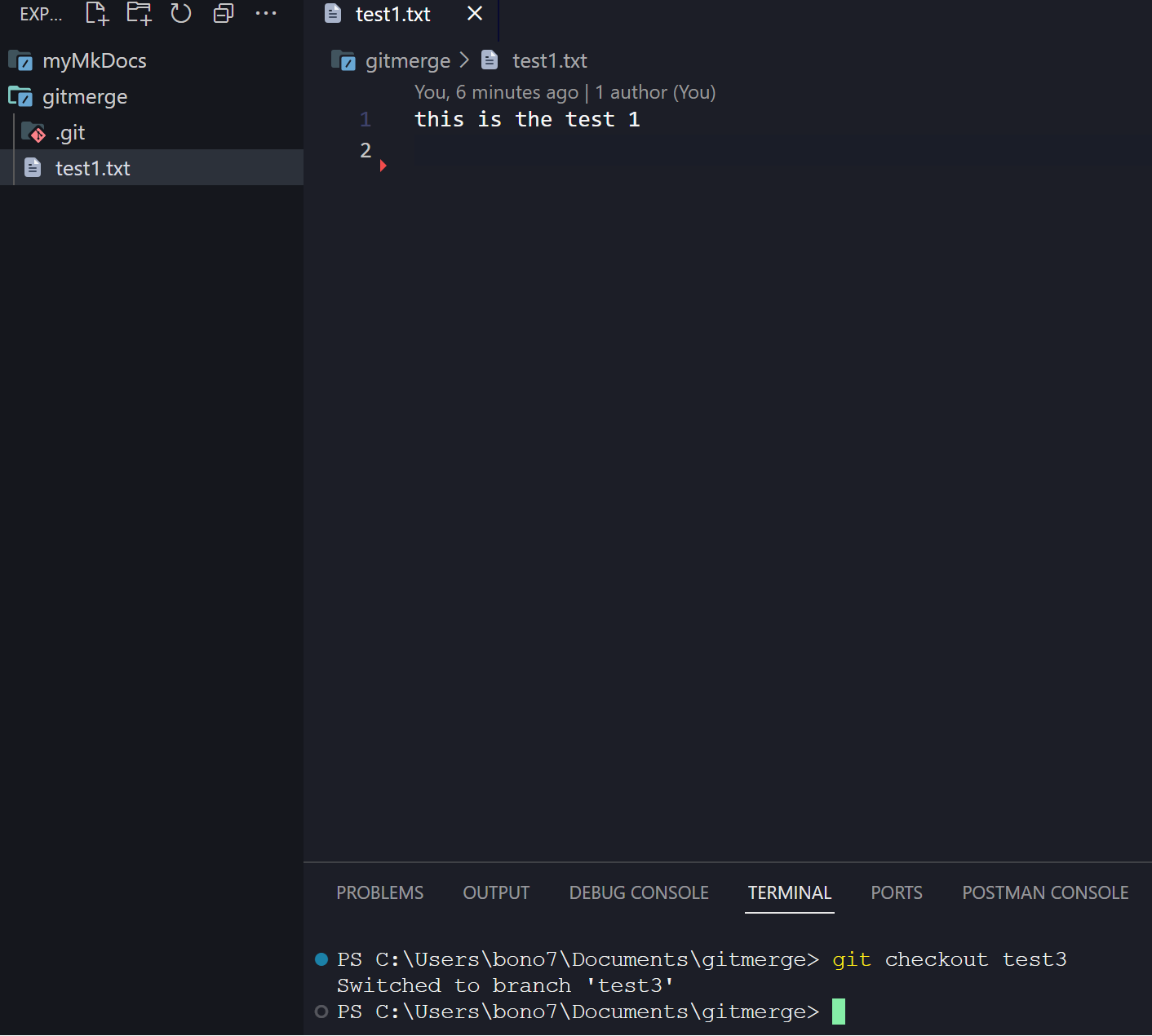
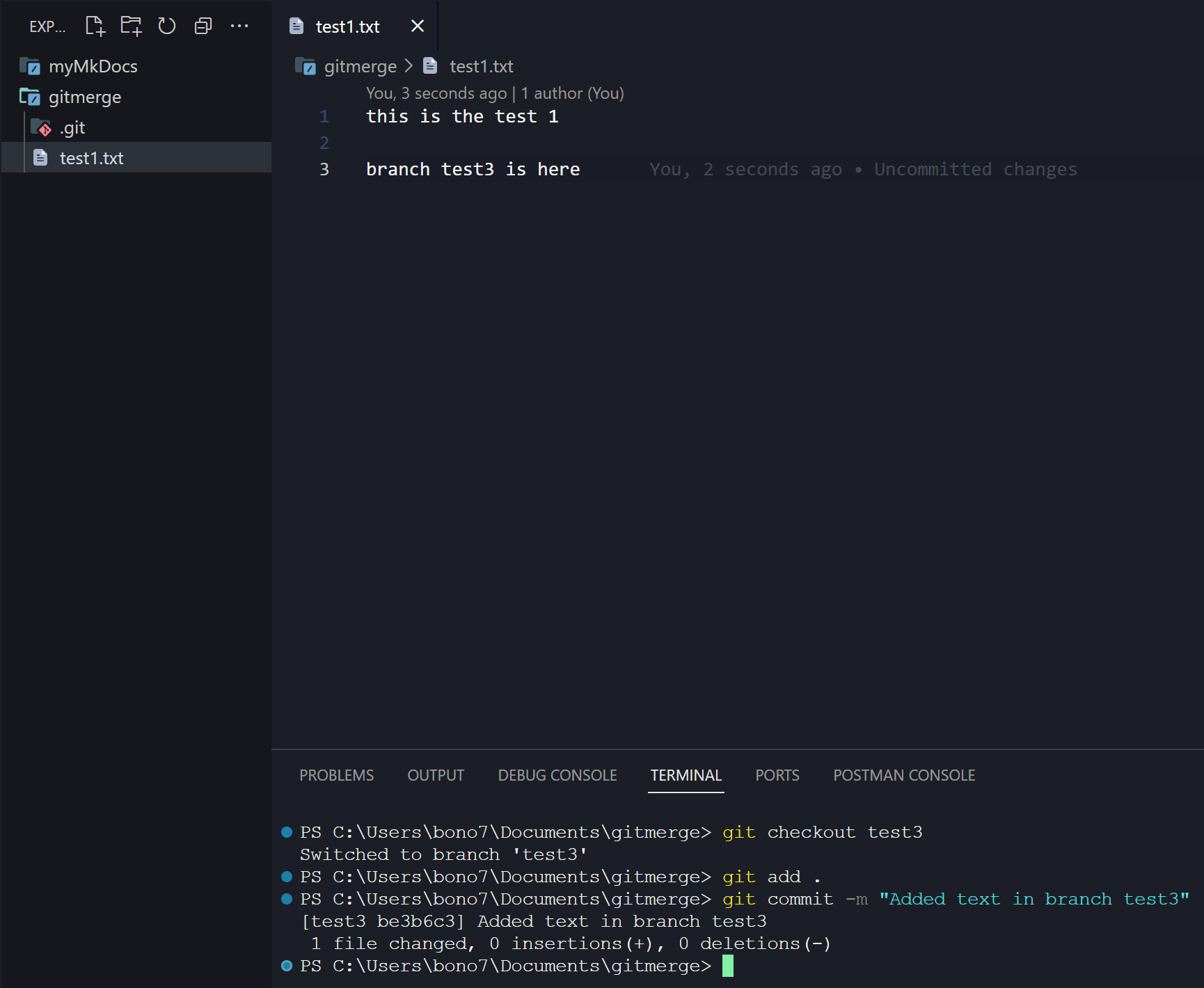
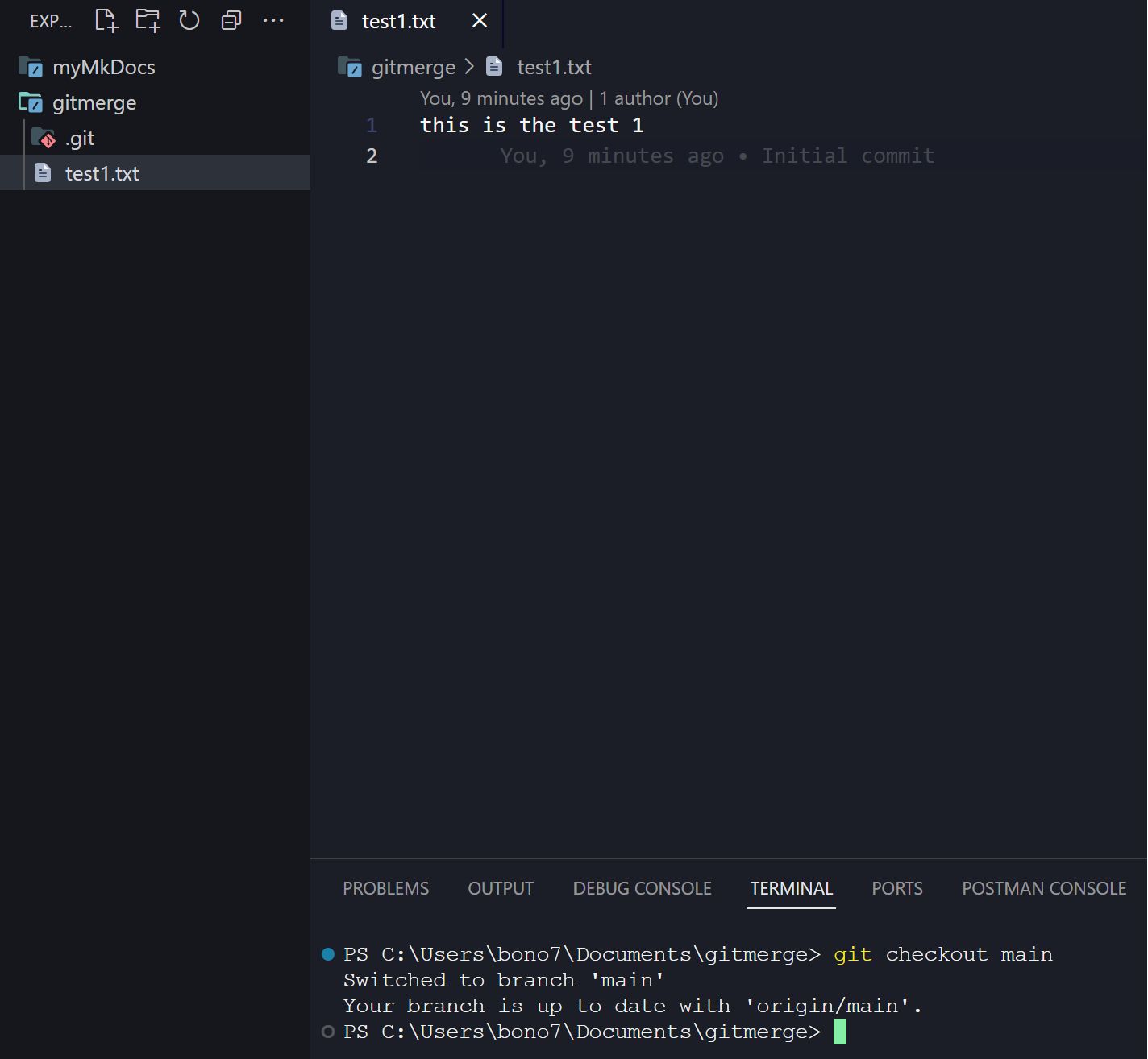
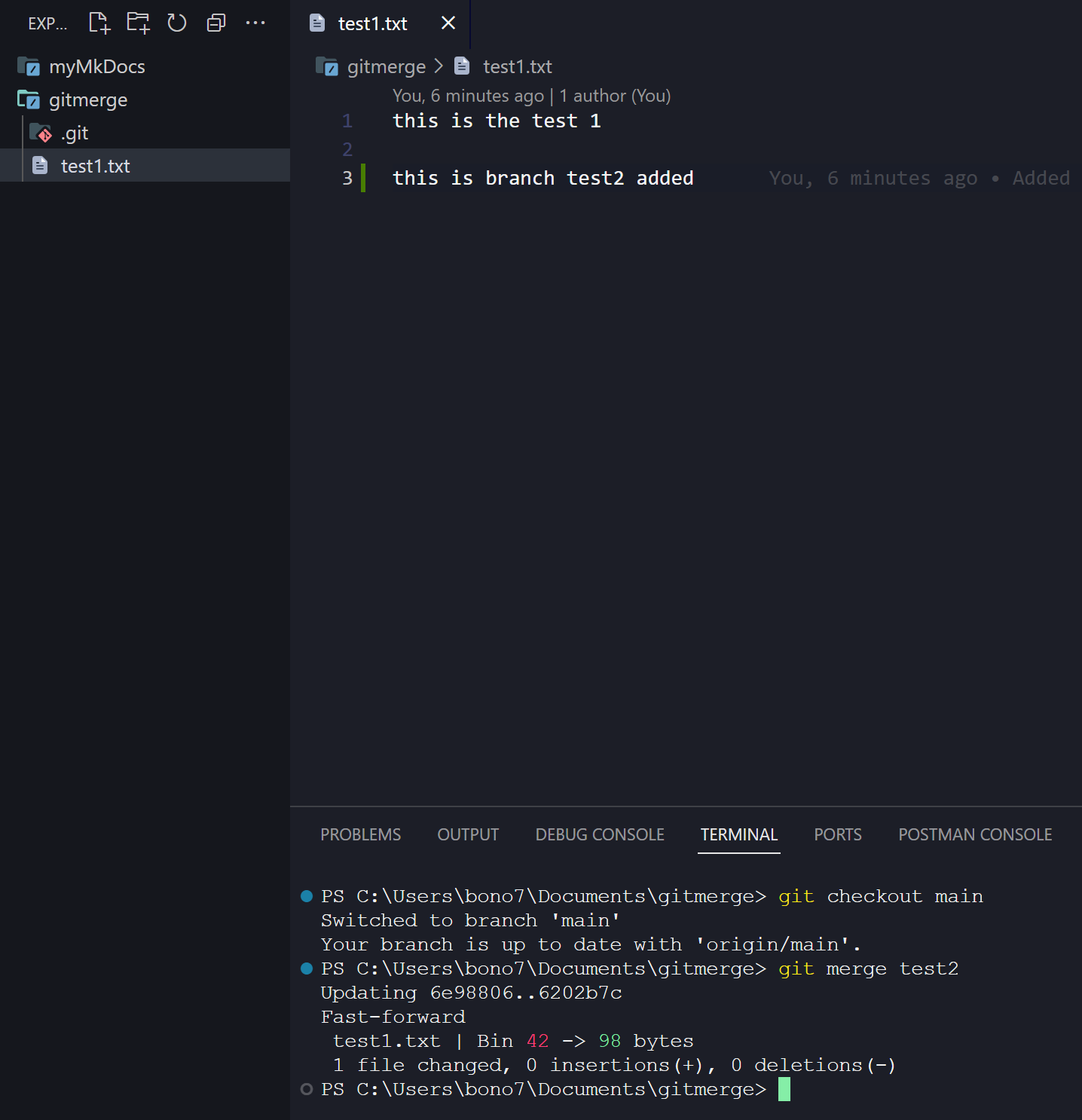
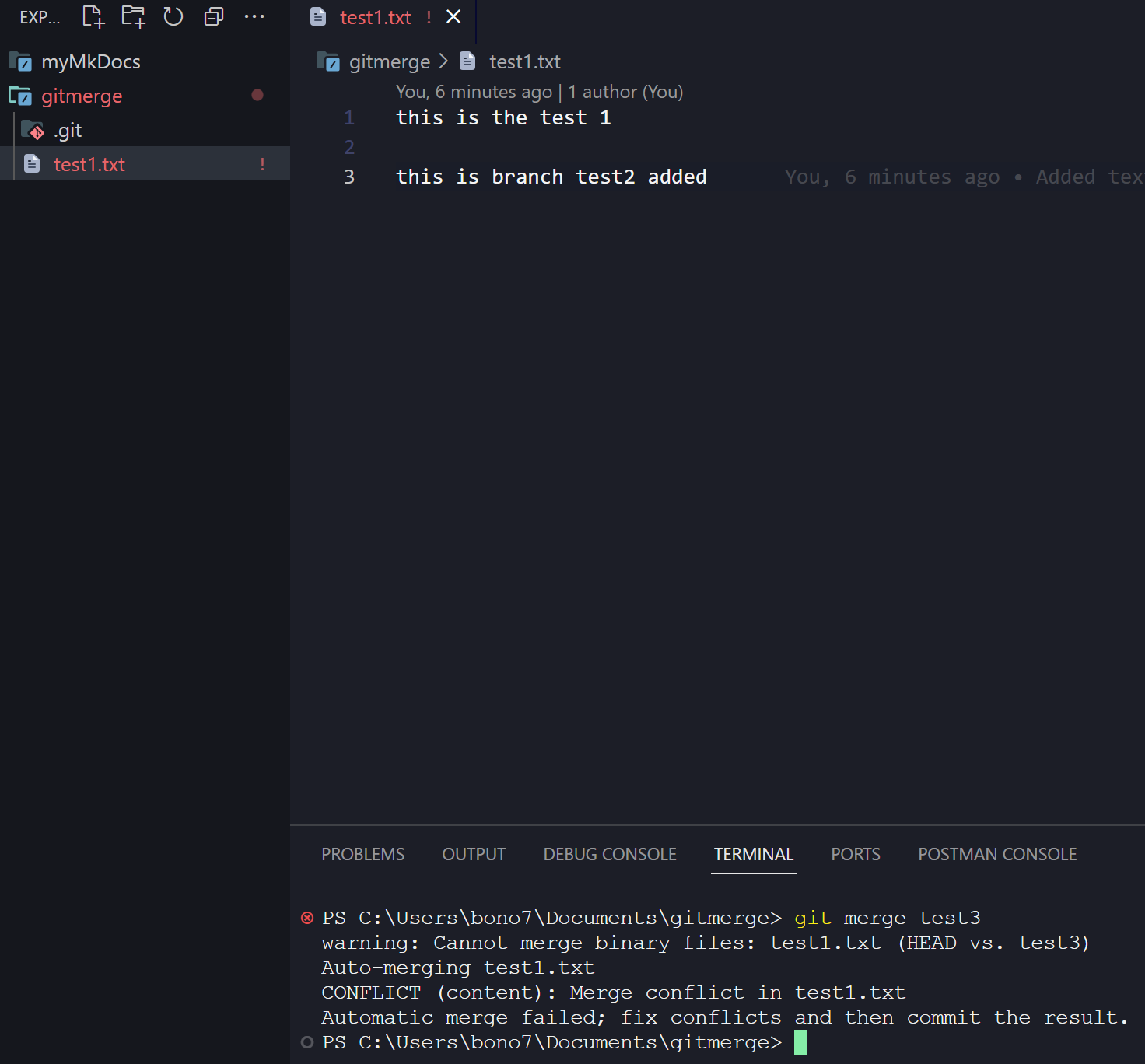
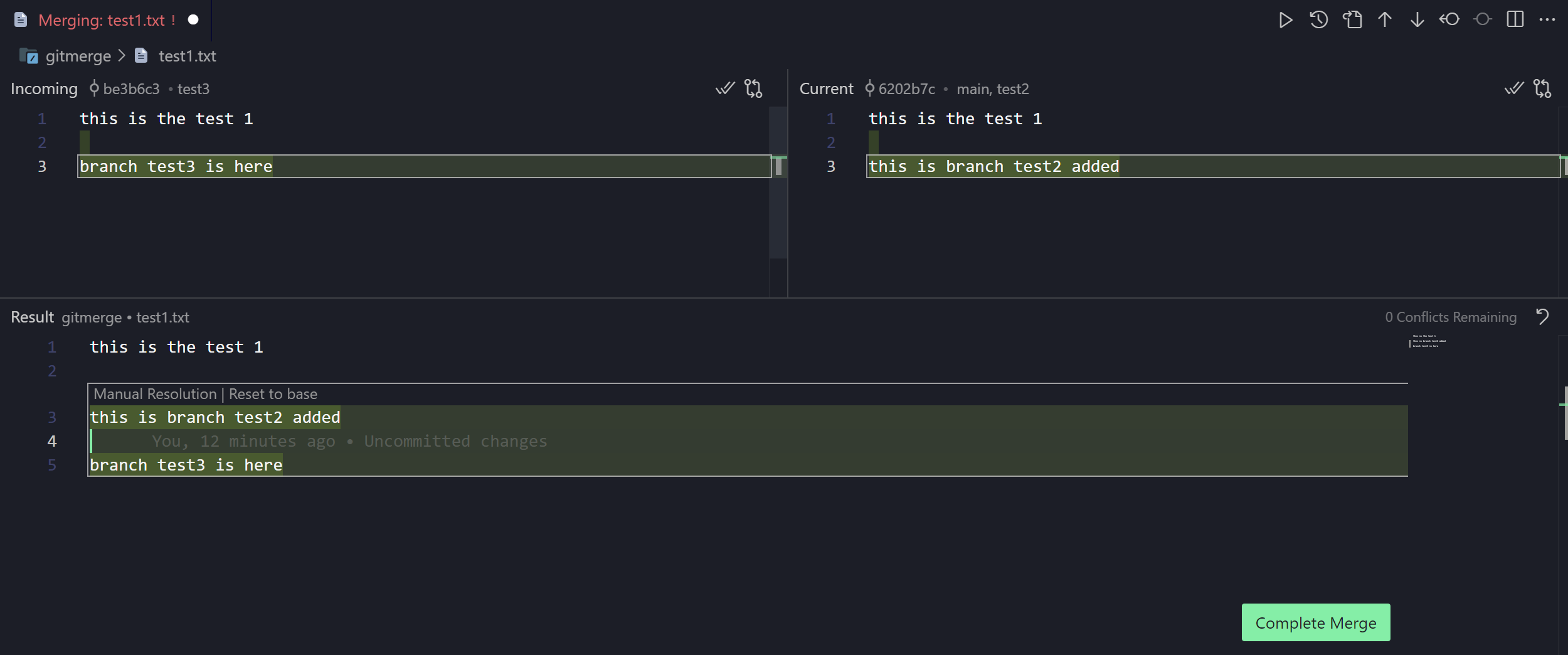
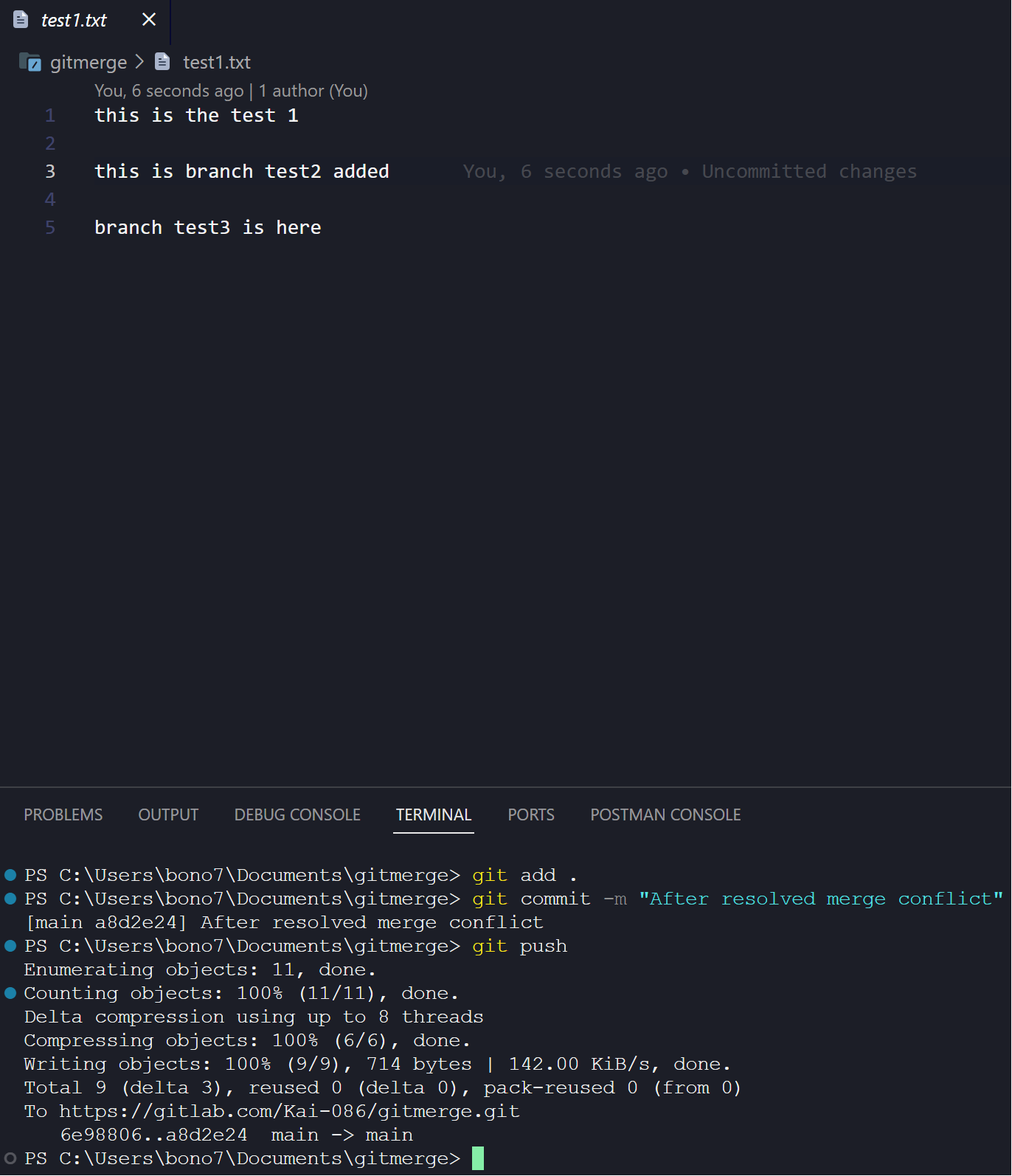
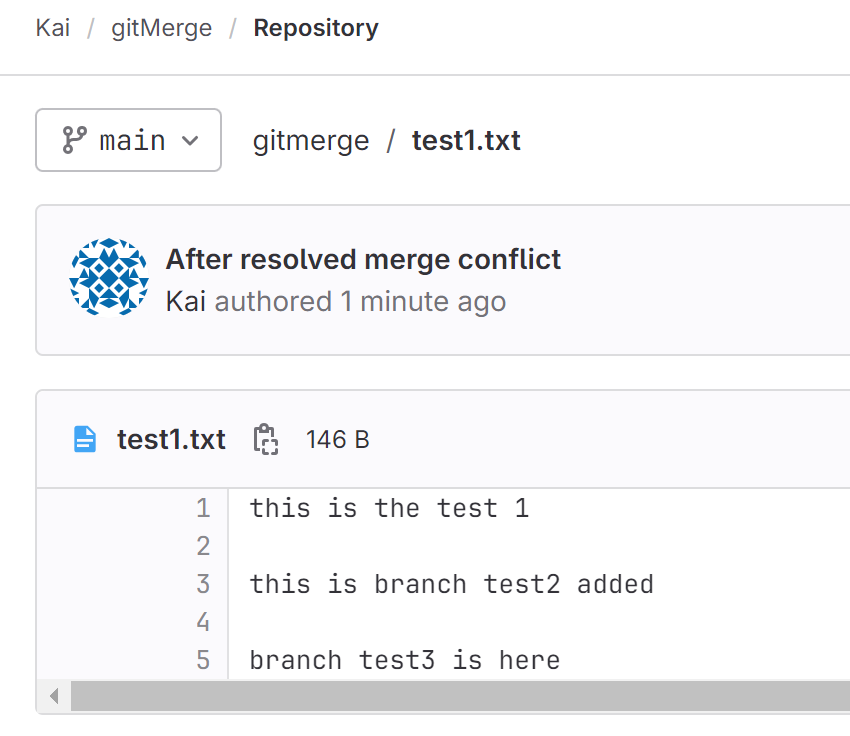
Merge Request#
Merge Request
Issue Tracking & Milestones#
Issues and Milestones
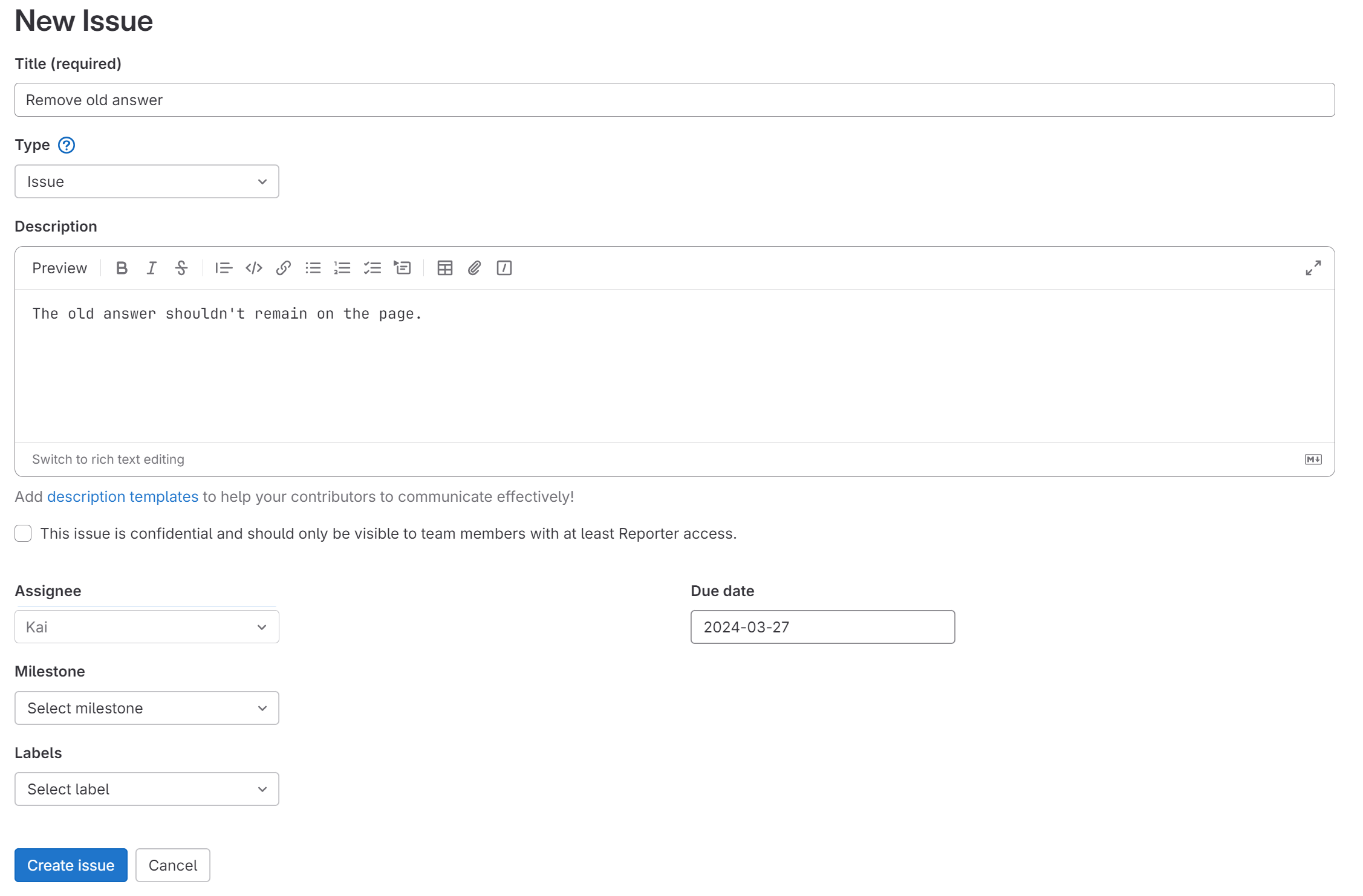
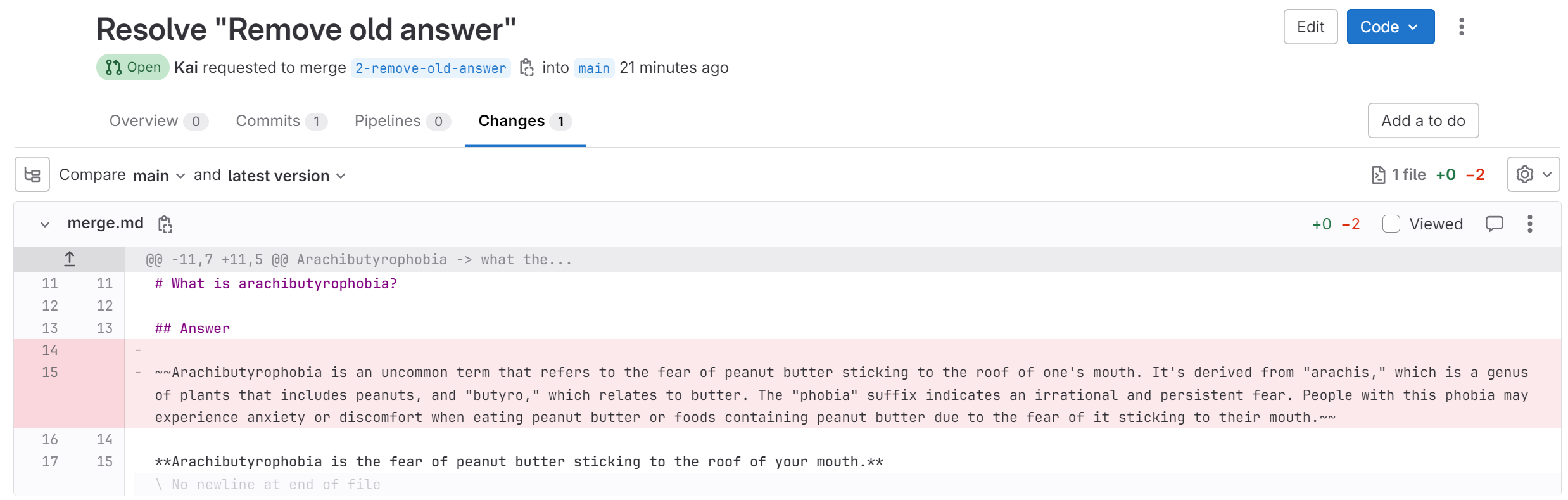
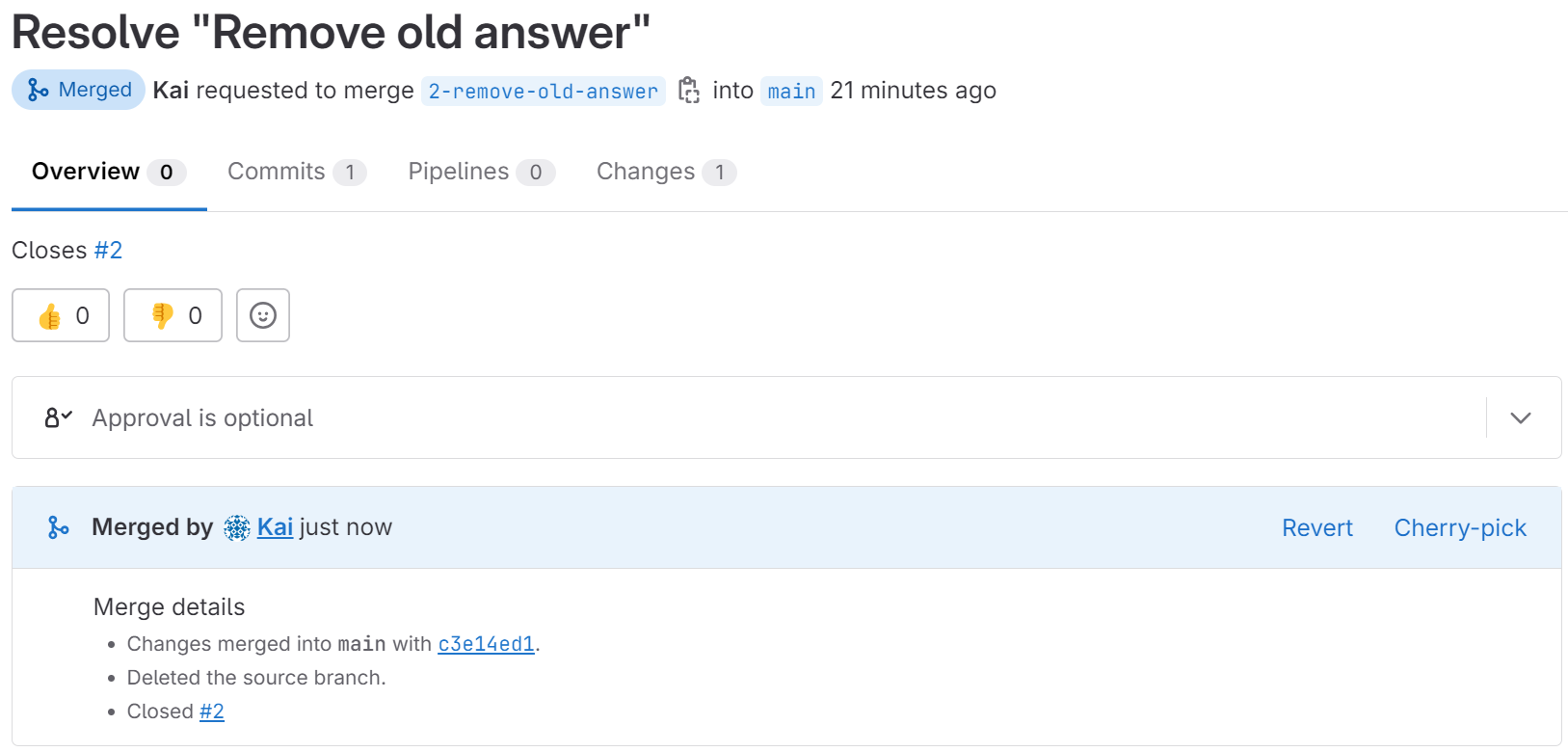
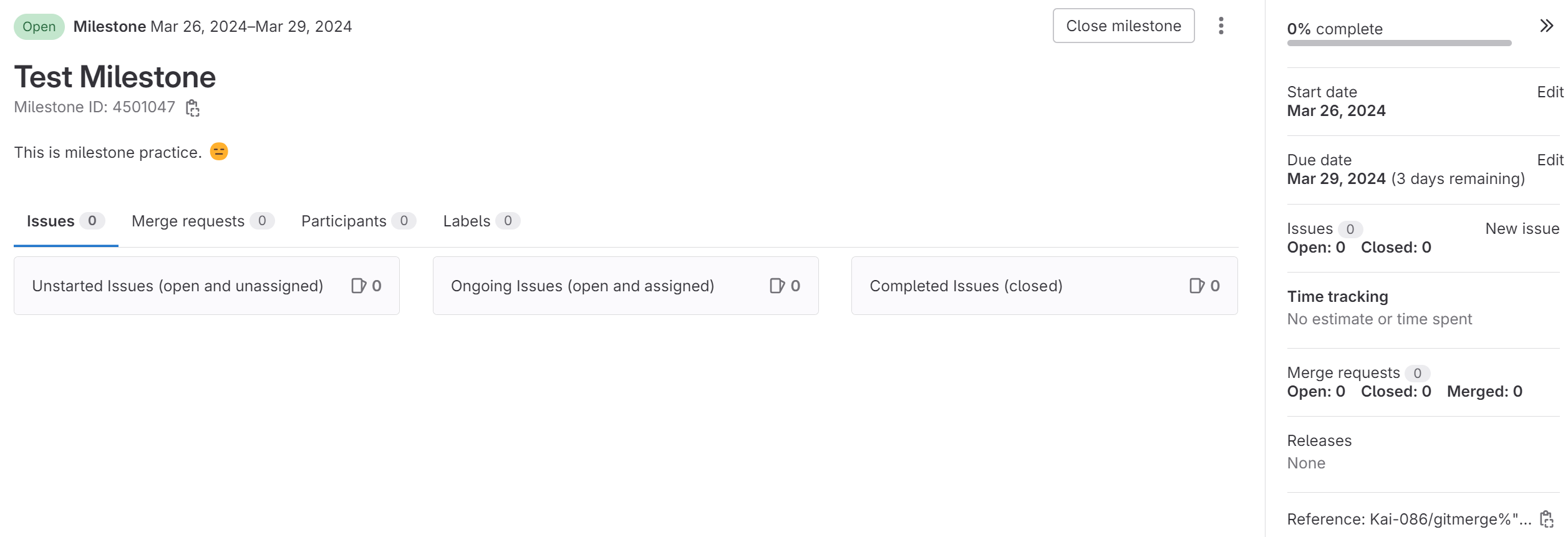
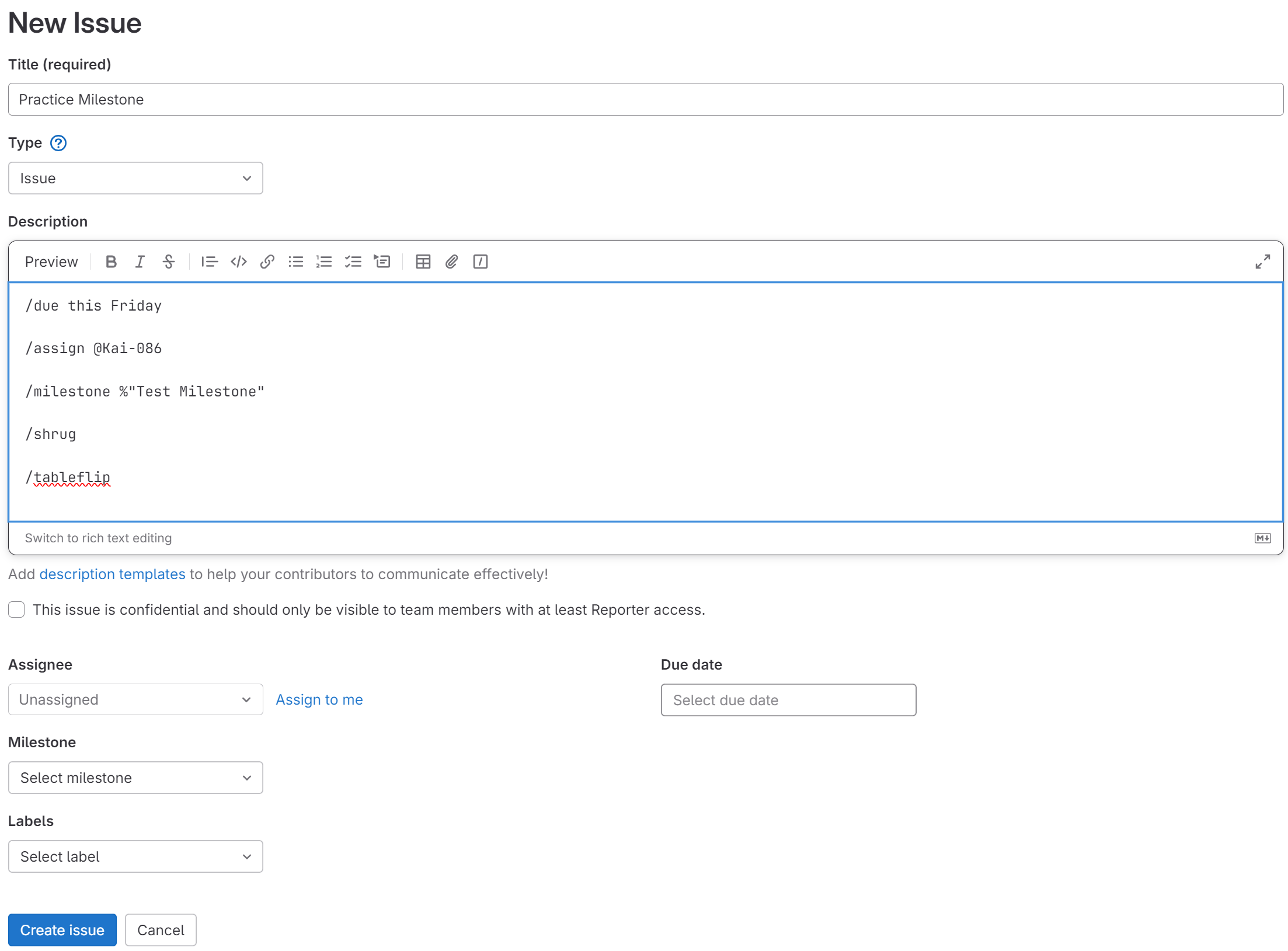
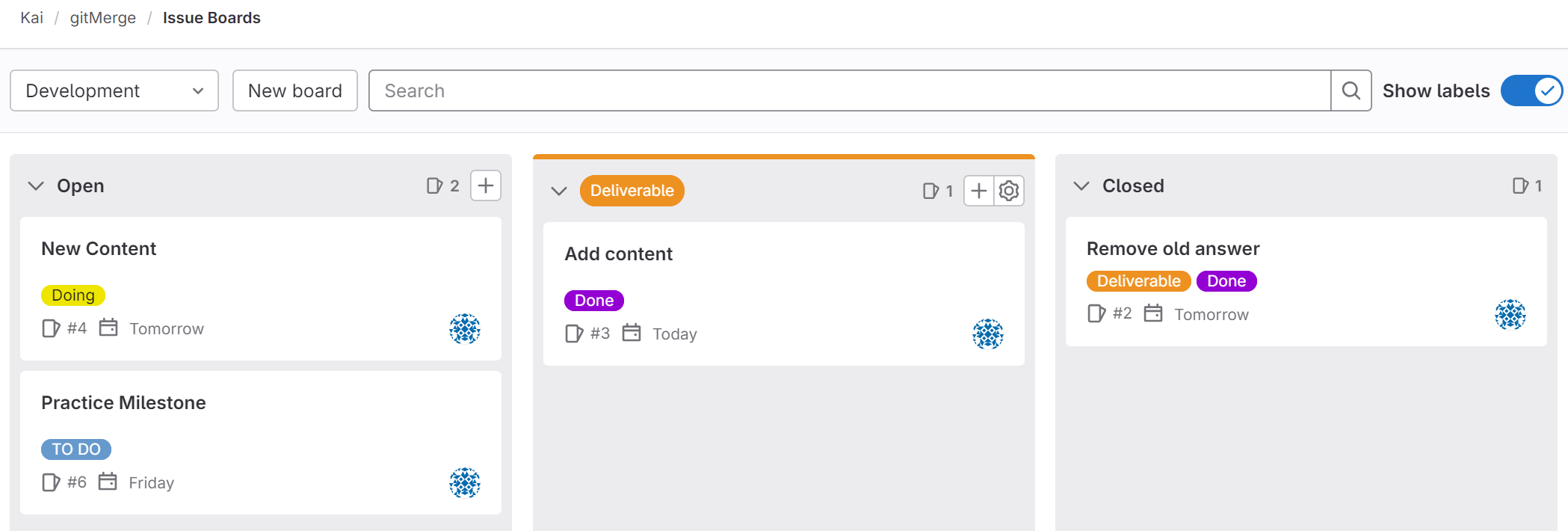
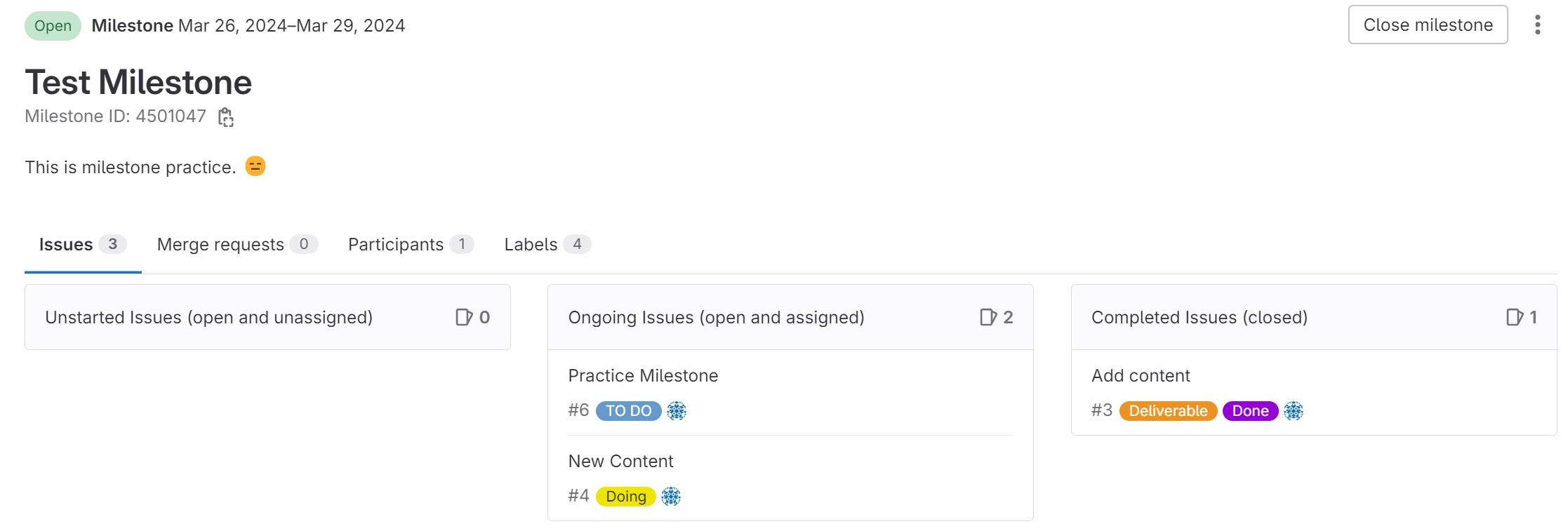
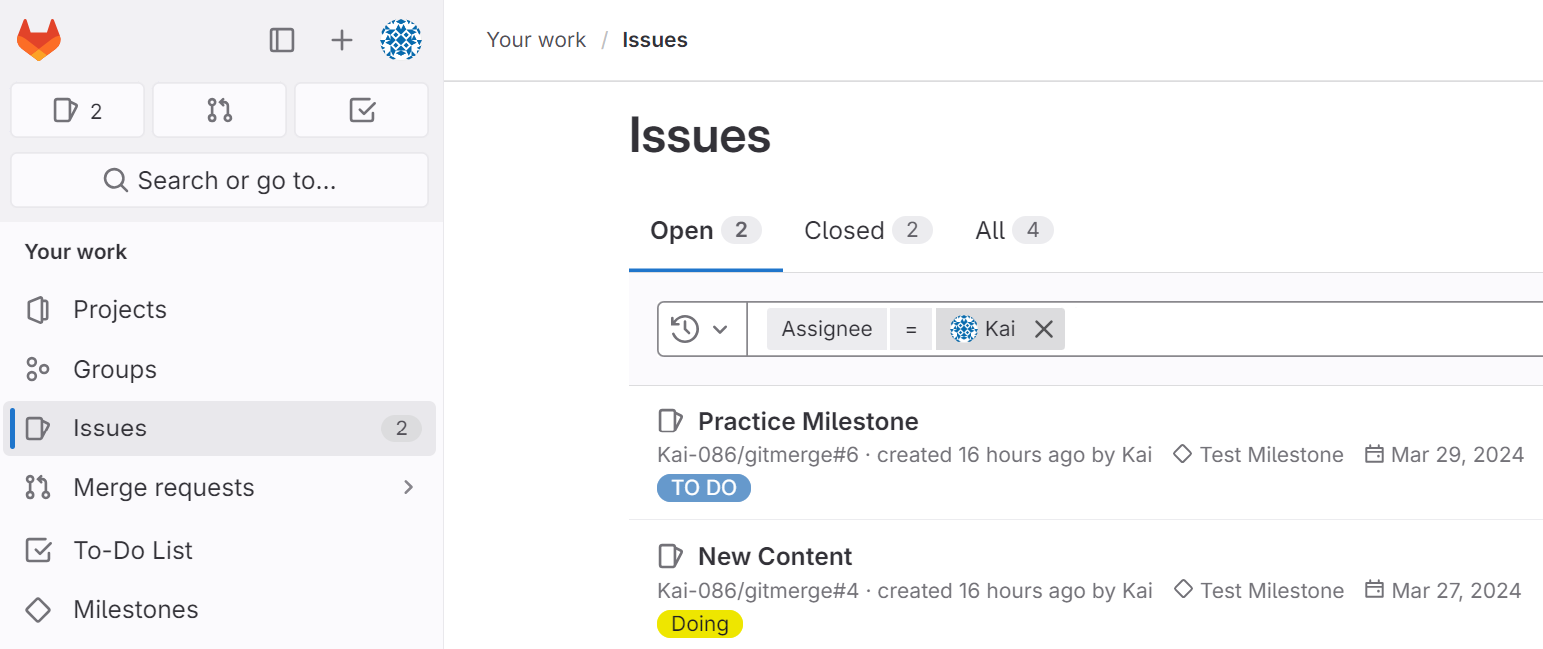
Ended: Git
JSON#
JavaScript Object Notation
Why use JSON Schema#
- Describe existing data format
- Define rules and constraints
- Clear and readable documentation
- Highly extensible
- Vaildate your data
- Automate testing
- Enhance data quality
- Wide range of tools availabiility
JSON Data Type#
-
Arrays
string, number, object, array, Boolean, or null
[ "first", 2, true, null ]
-
Boolean
true, false. Doesn't need
"
true false
-
Null
When no value is assigned to a key, it can be treated as null.
null
-
Number
Both positive and negative numbers as well as decimal points. A JSON numbers follows JavaScript's double-precision floating-point format (1).
-
- 64位元 IEEE 754浮點數
- 範圍: -21024 ~ +21023
465.3213
-
-
Ojbect
{ key1: value1, key2: value2 }
-
String
Strings are enclosed in
"
, can contain any Unicode (1) character.-
- 因為ASCII code不足以支持所有的語系,所以有了Unicode的誕生。
- UTF-8:
- Unicode Transformation Format (UTF)
- 可變長度的編碼,使用1到4個位元組來表達一個字元,並與ASCII相容
- 世界最廣泛的編碼方式
"This is a string"
-
JSON Schema#
- Data is name(key)/ value pairs
- Data is separated by commas
- Curly braces hold objects
- Square brackets hold arrays
Keywords#
Metadata keywords#
$schema
: states that this schema complies$id
: provides a base URI for referencing this schema, type, or propertytitle
: describes the intent of a schemadescription
: provides a definition for this type or property
Validation Keywords#
-
Numbers and Integers
multipleOf
: limit the value to be a multiple of a given numberminimum
,exclusiveMinimum
,maximum
,exclusiveMaxmum
: restrict the range of values
-
Strings
minLength
,maxLength
: limit the length of the stringpattern
: require the value to satisfy of the stringformat
: require the value to match a certain format
-
Arrays
type
: limit the type of each itemenum
: define the alloable values of each item with an arrayminItems
,maxItems
: limit the number or itemsuniqueItems
: require each item to be unique
-
Objects
properties
: list properties that may be included in the objectrequired
: list properties that must be included in the objectadditionalProperties
: allow properties that are not listed in the type definitionminProperties
,maxProperties
: limit the number of properties in the objectpatternProperties
: define types for properties based on their namedependencies
: add restrictions if certain conditions are met
allOf
: must be valid against all of the subschemasanyOf
: must be valid against any of the subschemas-
oneOf
: must be valid against exactly one of the subschemas -
$ref
,$def
: must be an object, and object must be a valid JSON schema
Create a Simple JSON Schema#
Differences#
JSON vs. XML vs. YAML
JSON#
- Commonly used for data storage and transfer.
XML (Extensible Markup Language)#
- It is general-purpose markup language similar to JSON that allows for more complex data structures.
YAML#
-
It was designed specifically to be easier for humans to read, and originally built to simplify XML.
- Indentation to represent objects
- Colons to separate key-value pairs
- Hyphens for arrays
- Hashes to denote a comment
References#
RxJS#
RxJS is a library for composing asynchronous and event-based programs by using observable sequences.
For#
- Asynchronous programming
- Stream
3 way to design#
- Observer pattern
- Iterator pattern
- Functional programming
Observables#
Can be observed, and target can be anything
3 types of notifications
- next
- error
- complete
Observer#
A consumer of values delivered by an Observable
Operators#
Two types of operator#
-
- Pipeable Operators
- Can be piped to Observables using the syntax.
-
- Creation Operators
- Can be called as standalone functions to create a new Observables.
Commend operators#
Operators
-
From official website ↩
TypeScript ↵
TypeScript#
什麼是 TypeScript#
TypeScript 是 Microsoft 開發的開放原始碼語言,它解決了 JavaScript 在大型應用程式上支援的不足。TypeScript 是 JavaScript 的 superset,代表說它具備 JavaScript 的功能,可以編譯成純 JavaScript 的程式碼,並且還多了一些功能,像是:
- Interfaces
- Generics
- Enums
- etc.
這些都使得開發者能夠更有效的開發大型專案,而 TypeScript 有幾點特色:
- Object-Oriented language
- static type checking
- ES6 features
- Type hints
- etc.
Functions and Classes#
Functions#
Function Declaration#
function sum(x: number, y: number): number {
return x + y;
};
console.log(`Declaration: ${sum(10, 10)}`);
Function Expression#
let sum2 = function (x: number, y: number): number {
return x + y;
};
console.log(`Expression: ${sum(20, 20)}`);
Arrow Function#
Used for anonymous functions
(param1,..., paramN) => expression
Used =>
, passed parameters in ()
, and expression enclosed within {}
without parameters:
Type#
Type annotation#
在參數後加上 : type
,先宣告型別,若賦予其他型別將會報錯。
藉由Type annotation執行靜態型別檢查,預防錯誤且可以統一規格,增加程式碼的可讀性。
Big Integer: 253 -1 ES2020
H2O
Ended: TypeScript
VS Code ↵
VS Code Extension#
VSCode Architecture#
Create Extension#
Install Yeoman and VS Code Extension Generator
-
Do not want to install Yeoman for later use:
-
Want to install Yeoman globally for repeated use:
-
What type of extension do you wnat to create?
建立 Extension 類型
option desc New Extension (TypeScript) Create a new extension using TypeScript New Extension (JavaScript) Create a new extension using JavaScript New Color Theme Create a new color theme New Language Support Define syntax highlighting rules and language-specific features Code Snippets Create custom code snippets Keymap Customize the keyboard shortcuts Extension Pack Bundle multiple VS Code extension Language Pack (Localization) Localize the UI and messages New Web Extension Leverage web technologies such as HTML, CSS, and JavaScript New Notebook Renderer (TypeScript) Create custom notebook renderer -
What's the name of your extension?
Extension 名稱
-
What's the identifier of your extension?
Extension 識別碼
-
What's the description of your extension?
描述
-
Initialize a git repository?
要Git? 預設: Y
-
Bundle the source code with webpack?
要使用 webpack 打包原始碼? 預設: N
-
Which package manager to use?
要使用哪個套件管理工具
1. npm
2. yarn
3. pnpm -
Do you want to open the new folder with Visual Studio Code?
npm, yarn, and pnpm#
-
- npm (Node Package Manager)
-
JavaScript套件管理工具,讓使用者可以輕鬆安裝、共享和管理JS的library
- 優點:
- 大量的模組
- 與 GitHub 無縫集成
- 活躍的社群支持
- 穩定
- 缺點:
- 安裝時間較慢
- 有依賴性衝突的可能
-
- yarn
-
改善 npm 的缺點,且確保可以在不同的設備上進行一致的安裝
- 優點:
- 安裝較快速
- 使用 yarn.lock 進行一致的安裝
- 用於管理多儲存庫的工作區
- 缺點:
- 相較於 npm,需要些時間學習
- 不是所有 npm 模組都兼容
-
- pnpm
-
解決 npm 與 yarn 在某些情況會遇到的問題,特別是與 node_modules 大小有關
- 優點:
- 高效的硬碟空間使用
- 比 yarn 更快的安裝
- 一致且可重複的夠建
- 缺點:
- 沒有像 npm 或 yarn 一樣被廣泛使用
npm yarn pnpm 速度 儲存 安全 相容性 熱門程度 使用 package-lock.json yarn.lock pnpm-lock.yaml
npx#
npx 是 npm v5.2.0 開始加入的指令,與 npm 的差別在於
npm => 永久存在於主機
npx => 臨時安裝並在執行完後刪除
npx 的功能:
- 直接執行二進制文件
- 運行未全局安裝的套件
- 從 URL 執行代碼
- 測試不同版本的套件
Extension Structure#
.
|-- .vscode
| |-- launch.json // Config fro launching and debugging the extension
| |-- tasks.json // Config for build task that compiles TypeScript
|-- .gitignore // Ignore build output and node_modules
|-- README.md // Readable description of your extension's functionality
|-- src
| |-- extension.ts // Extension source code
|-- package.json // Extension manifest
|-- tsconfig.json // TypeScript configuration
package.json#
{
"name": "testextension", // 名稱
"displayName": "testExtension", // 顯示的名稱
"description": "my test extension practice", // 描述
"version": "0.0.1", // 版本
"engines": { // 最低支持的VS Code API 版本
"vscode": "^1.88.0"
},
"categories": [
"Other"
],
"activationEvents": [ // 事件列表
"*" // onLanguage => onLanguage:python
// onCommand => 使用命令時啟動
// * => 一啟動vscode就啟動
],
"main": "./out/extension.js", // extension 進入點
"contributes": {
"commands": [ // 命令列表
{
"command": "testextension.helloWorld",
"title": "Hello World"
}
]
},
"scripts": {
"vscode:prepublish": "npm run compile",
"compile": "tsc -p ./",
"watch": "tsc -watch -p ./",
"pretest": "npm run compile && npm run lint",
"lint": "eslint src --ext ts",
"test": "vscode-test"
},
"devDependencies": {
"@types/vscode": "^1.88.0",
"@types/mocha": "^10.0.6",
"@types/node": "18.x",
"@typescript-eslint/eslint-plugin": "^7.4.0",
"@typescript-eslint/parser": "^7.4.0",
"eslint": "^8.57.0",
"typescript": "^5.3.3",
"@vscode/test-cli": "^0.0.8",
"@vscode/test-electron": "^2.3.9"
}
}
Run Extension#
extension.ts#
// The module 'vscode' contains the VS Code extensibility API
// Import the module and reference it with the alias vscode in your code below
import * as vscode from 'vscode';
// This method is called when your extension is activated
// Your extension is activated the very first time the command is executed
export function activate(context: vscode.ExtensionContext) {
// Use the console to output diagnostic information (console.log) and errors (console.error)
// This line of code will only be executed once when your extension is activated
console.log('Congratulations, your extension "testextension" is now active!');
// The command has been defined in the package.json file
// Now provide the implementation of the command with registerCommand
// The commandId parameter must match the command field in package.json
let disposable = vscode.commands.registerCommand('testextension.helloWorld', () => {
// The code you place here will be executed every time your command is executed
// Display a message box to the user
vscode.window.showInformationMessage('Hello World from testExtension!');
});
context.subscriptions.push(disposable);
}
// This method is called when your extension is deactivated
export function deactivate() {}
Run and Debug#
subscription 為 extension 定用命令且確保 extension 在 unloaded 後會解除註冊
Extension Development Host#
References#
- Your First Extension
- vscode-extension-samples
- 自己用的工具自己做! 30天玩轉VS Code Extension之旅
- Let’s build an Extention for Visual Studio Code(VScode)
- npm vs yarn vs pnpm,誰是你的最佳選擇?
- Comparing NPM, YARN, and PNPM Package Managers: Which One is Right for Your Distributed Project to handle High Loads?
- [NodeJS] npx 是什麼? 跟 npm 差在哪?
Extension Hands On#
@https://github.com/microsoft/vscode-extension-samples
Command#
Usage#
@https://code.visualstudio.com/api/references/vscode-api
API | Desc | |
---|---|---|
executeCommand |
Executes the command denoted by the given command identifier | 透過給定的 command id 執行對應的 command |
getCommands(filterInternal?: boolean) | Retrieve the list of all available commands. Commands starting with an underscore are treated as internal commands | 取得 command ,可以給個 boolean 值決定使否列出vscode內部使用的 command |
registerCommand(command: string, callback) | Registers a command that can be invoked via a keyboard shortcut, a menu item, an action, or directly | 在 vscode 中註冊一個可以被 keyboard shortcut、extension 或其他 UI 元件呼叫的命令 |
registerTextEditorCommand(command: string, callback) | Registers a text editor command that can be invoked via a keyboard shortcut, a menu item, an action, or directly | 跟 register 相似,但只有在 vscode 開啟文件的 editor 時才會觸發 |
Code & Detail#
Command API
Context Menu#
Usage#
@https://code.visualstudio.com/api/ux-guidelines/context-menus
@https://code.visualstudio.com/api/references/contribution-points#contributes.menus
{
"contributes": {
"menus": {
"${extension writers can contribute to}": [
{
"alt": "${commandID}",
"command": "${commandID}",
"group": "${sorting group}",
"submenu": "${submentID}",
"when": "${boolean}",
}
]
}
}
}
contributes | desc |
---|---|
alt | identifier of an alternative command to execute |
command | identifier of the command to execute |
group | group into which this item belongs |
submenu | identifier of the submenu to display in this item |
when | condition which must be true to show this item |
Code & Detail#
Context Menu
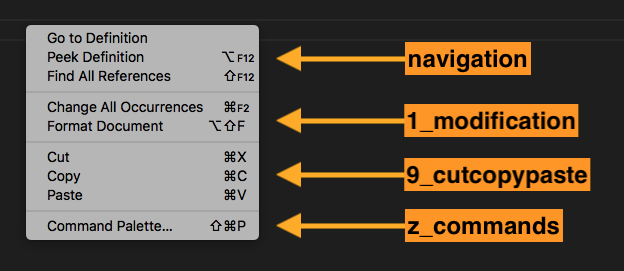
TreeView, DataProvider & View Container#
TreeView
View Container
Usage#
@https://code.visualstudio.com/api/extension-guides/tree-view
TreeView#
identifier | desc |
---|---|
explorer | explorer view in the side bar |
debug | run and debug view in the side bar |
scm | source control view in the side bar |
test | test explorer view in the side bar |
TreeDataProvider#
TreeDataProvider | desc | |
---|---|---|
getTreeItem | return the children for the given element or root (if no element is passed) | 回傳TreeView呈現的TreeItem Element |
getChildren | return the UI representation (TreeItem) of the element that gets displayed in the view | 回傳TreeView底下的treeItem們 |
Registering | desc | |
---|---|---|
vscode.window.registerTreeDataProvider | register the tree data provider by providing registered view ID and data provider | |
vscode.window.createTreeView | create the Tree View by broviding view ID and data provider |
Updating content | desc | |
---|---|---|
onDidChangeTreeData | if your tree data can change and you want to update the treeview | 使用EventEmitter的event監聽方法,通知DataProvider的訂閱者資料已經發生變化 |
View Container#
fields | |
---|---|
id | The ID of the new view container |
title | The name that will show up the top of the veiw container |
icon | An image that will be displayed for the view container when in the Activity Bar |
Code & Detail#
TreeView & DataProvider
View Container & DataProvider
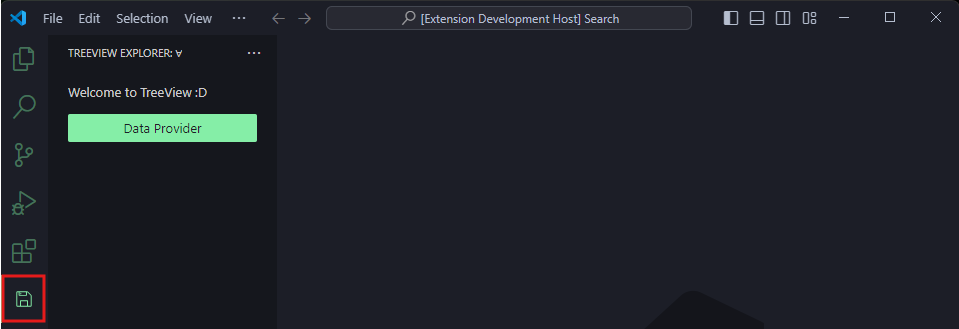
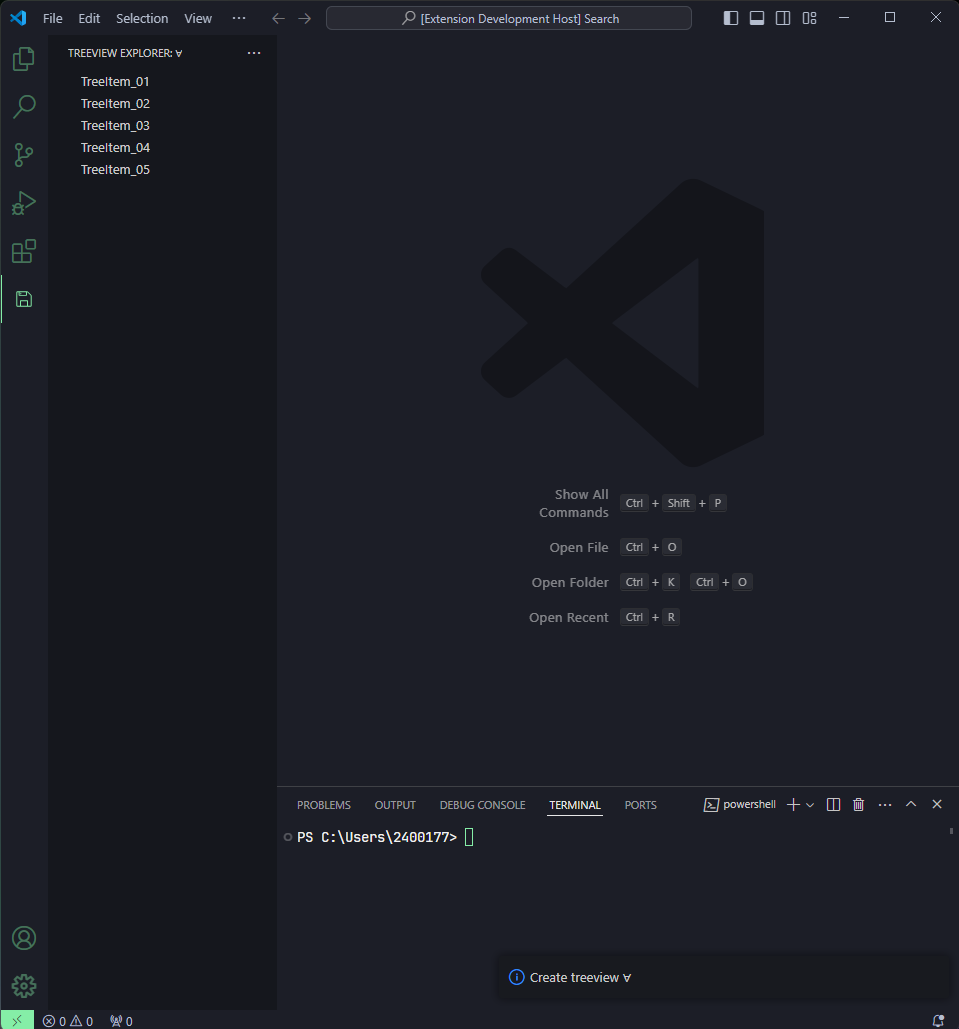
Output Channel#
Usage#
@https://code.visualstudio.com/api/references/vscode-api#OutputChannel
property | desc |
---|---|
name: string | The human-readable name of this output channel |
methods | desc |
---|---|
append | A string, falsy values will not be printed |
appendLine | A string, false values will not be printed |
clear | Removes all output from the channel |
dispose | Dispose and free associated resources |
hide | Hide this channel from the UI |
replace | Replaces all output from the channel with the given value |
show | Reveal this channel in the UI |
Code & Detail#
Output Channel
Workspace API#
Usage#
@https://code.visualstudio.com/api/references/contribution-points#contributes.configuration
Configuration schema | desc |
---|---|
The heading used for that category | |
Dictionary of configuration properties | |
After the title and before the input field, except for booleans, where the description is used as the label for the checkbox | |
Can be edited directly in the setting UI | |
Provides descriptive text rendered at the bottom of the dropdown |