Extension Hands On#
@https://github.com/microsoft/vscode-extension-samples
Command#
Usage#
@https://code.visualstudio.com/api/references/vscode-api
API | Desc | |
---|---|---|
executeCommand |
Executes the command denoted by the given command identifier | 透過給定的 command id 執行對應的 command |
getCommands(filterInternal?: boolean) | Retrieve the list of all available commands. Commands starting with an underscore are treated as internal commands | 取得 command ,可以給個 boolean 值決定使否列出vscode內部使用的 command |
registerCommand(command: string, callback) | Registers a command that can be invoked via a keyboard shortcut, a menu item, an action, or directly | 在 vscode 中註冊一個可以被 keyboard shortcut、extension 或其他 UI 元件呼叫的命令 |
registerTextEditorCommand(command: string, callback) | Registers a text editor command that can be invoked via a keyboard shortcut, a menu item, an action, or directly | 跟 register 相似,但只有在 vscode 開啟文件的 editor 時才會觸發 |
Code & Detail#
Command API
Context Menu#
Usage#
@https://code.visualstudio.com/api/ux-guidelines/context-menus
@https://code.visualstudio.com/api/references/contribution-points#contributes.menus
{
"contributes": {
"menus": {
"${extension writers can contribute to}": [
{
"alt": "${commandID}",
"command": "${commandID}",
"group": "${sorting group}",
"submenu": "${submentID}",
"when": "${boolean}",
}
]
}
}
}
contributes | desc |
---|---|
alt | identifier of an alternative command to execute |
command | identifier of the command to execute |
group | group into which this item belongs |
submenu | identifier of the submenu to display in this item |
when | condition which must be true to show this item |
Code & Detail#
Context Menu
group sorting
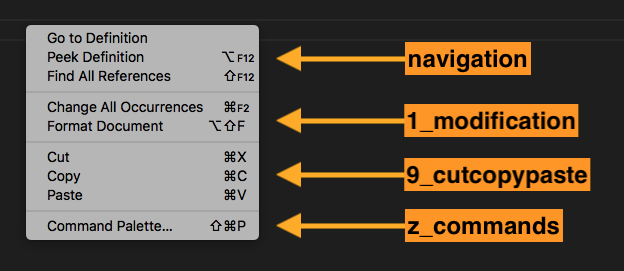
TreeView, DataProvider & View Container#
TreeView
View Container
Usage#
@https://code.visualstudio.com/api/extension-guides/tree-view
TreeView#
identifier | desc |
---|---|
explorer | explorer view in the side bar |
debug | run and debug view in the side bar |
scm | source control view in the side bar |
test | test explorer view in the side bar |
TreeDataProvider#
TreeDataProvider | desc | |
---|---|---|
getTreeItem | return the children for the given element or root (if no element is passed) | 回傳TreeView呈現的TreeItem Element |
getChildren | return the UI representation (TreeItem) of the element that gets displayed in the view | 回傳TreeView底下的treeItem們 |
Registering | desc | |
---|---|---|
vscode.window.registerTreeDataProvider | register the tree data provider by providing registered view ID and data provider | |
vscode.window.createTreeView | create the Tree View by broviding view ID and data provider |
Updating content | desc | |
---|---|---|
onDidChangeTreeData | if your tree data can change and you want to update the treeview | 使用EventEmitter的event監聽方法,通知DataProvider的訂閱者資料已經發生變化 |
View Container#
fields | |
---|---|
id | The ID of the new view container |
title | The name that will show up the top of the veiw container |
icon | An image that will be displayed for the view container when in the Activity Bar |
Code & Detail#
TreeView & DataProvider
View Container & DataProvider
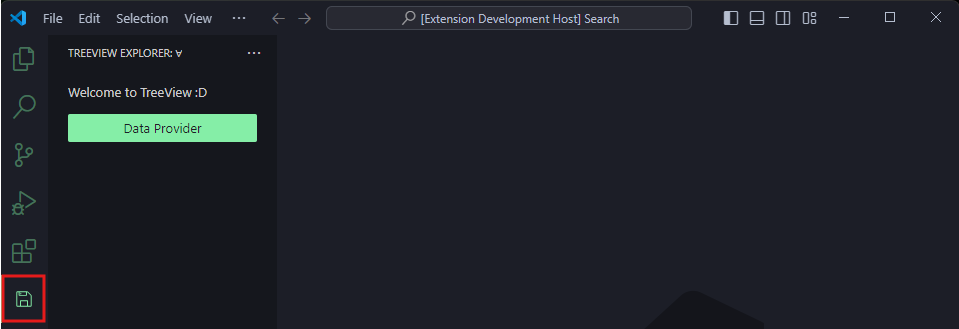
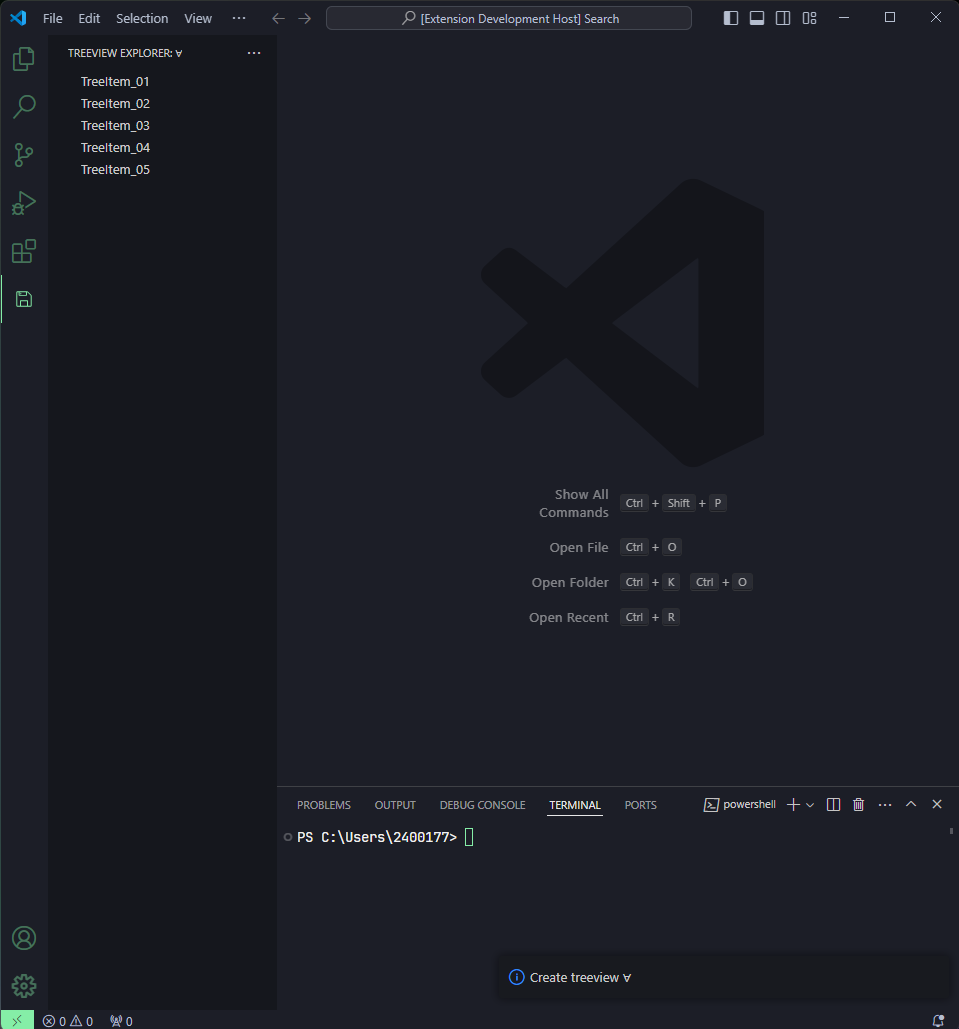
Output Channel#
Usage#
@https://code.visualstudio.com/api/references/vscode-api#OutputChannel
property | desc |
---|---|
name: string | The human-readable name of this output channel |
methods | desc |
---|---|
append | A string, falsy values will not be printed |
appendLine | A string, false values will not be printed |
clear | Removes all output from the channel |
dispose | Dispose and free associated resources |
hide | Hide this channel from the UI |
replace | Replaces all output from the channel with the given value |
show | Reveal this channel in the UI |
Code & Detail#
Output Channel
Workspace API#
Usage#
@https://code.visualstudio.com/api/references/contribution-points#contributes.configuration
Configuration schema | desc |
---|---|
The heading used for that category | |
Dictionary of configuration properties | |
After the title and before the input field, except for booleans, where the description is used as the label for the checkbox | |
Can be edited directly in the setting UI | |
Provides descriptive text rendered at the bottom of the dropdown |